React SDK
Crater React SDK is a library of ready-to-use React components. These components can help you embed invoicing and bill pay functionality into your React-based web application with just a few lines of code.
Works with:
Installation
Step 1: Install the Crater React SDK via npm or yarn
The Crater React SDK package is available on the NPM and Yarn directories. To install:
npm install @craterapp/react-sdk
Step 2: Configure CraterSDK
instance
The CraterSDK instance provides configuration for all Crater React UI components through the CraterProvider wrapper.
JavaScript
const crater = new CraterSDK({
tenantUrl: 'https://payments.your-domain.com', // We will provide you with the API URL to use
});
Properties
- Name
tenantUrl
- Type
- string
- Field Type
- Description
The URL for the API in use.
Step 3: Set Crater access token
In order to authorise the SDK components to access the Crater API, you need to first generate an access token from your backend. Once you have the access token, you need to call setToken
method to save the access token in the SDK.
You can view our Authentication documentation for more information on how to generate a new access token. The second param is the current business ID to scope the component to a specific business.
JavaScript
import { authService } from '@craterapp/react-sdk'
authService.setToken(`YOUR_ACCESS_TOKEN`, `YOUR_BUSINESS_ID`)
Step 4: Configure CraterProvider
Wrap your root component with CraterProvider.
The CraterProvider is the root component that must wrap all other Crater-connected components. The wrapper provides configuration for all Crater components beneath it.
App.tsx
<CraterProvider crater={crater} locale="en">
<div className="App">...</div>
</CraterProvider>
Step 5: Import Components and Styles
Import the components you want to use from the Crater React SDK package and the stylesheet. Any other components imported from @craterapp/react-sdk must be placed inside the CraterProvider
provider.
App.tsx
import { CraterProvider, CraterSDK, InvoiceTable } from '@craterapp/react-sdk'
import '@craterapp/react-sdk/dist/style.css'
return (
<CraterProvider crater={crater} locale="en">
<InvoiceTable />
</CraterProvider>
)
Step 6: Review implementation
If you followed the above steps correctly, your code should look like this:
App.tsx
import { CraterProvider, CraterSDK, InvoiceTable } from '@craterapp/react-sdk'
import { authService } from '@craterapp/react-sdk'
import '@craterapp/react-sdk/dist/style.css'
function App() {
useEffect(() => {
fetchCraterToken()
}, [])
const fetchCraterToken = () => {
fetch(`https://your-backend-api.com/access-token`).then((response) => {
authStore.setToken(response.token, response.businessId)
})
}
const crater = new CraterSDK({
tenantUrl: 'https://payments.your-domain.com', // We will provide you with the TENANT URL to use
})
return (
<CraterProvider crater={crater} locale="en">
<InvoiceTable />
</CraterProvider>
)
}
export default App
Step 7: Run the application
npm run start
Theme Config
You can use your own theme colors by updating the primary CSS variables inside :root
selector.
style.css
:root {
--cr-color-primary-50: 247, 246, 253;
--cr-color-primary-100: 238, 238, 251;
--cr-color-primary-200: 213, 212, 245;
--cr-color-primary-300: 188, 185, 239;
--cr-color-primary-400: 138, 133, 228;
--cr-color-primary-500: 88, 81, 216;
--cr-color-primary-600: 79, 73, 194;
--cr-color-primary-700: 53, 49, 130;
--cr-color-primary-800: 40, 36, 97;
--cr-color-primary-900: 26, 24, 65;
}
You can use the Tailwind Shades Generator to generate your own color theme. Just make sure to change the css color variable names correctly as given above after you copy the theme from the shades generator.
Advanced Customization
The above section outlines the primary color palette variables, but you can also modify all the other color variables mentioned below if needed.
style.css
:root {
--cr-color-gray-50: 248, 250, 252;
--cr-color-gray-100: 241, 245, 249;
--cr-color-gray-200: 226, 232, 240;
--cr-color-gray-300: 203, 213, 225;
--cr-color-gray-400: 148, 163, 184;
--cr-color-gray-500: 100, 116, 139;
--cr-color-gray-600: 71, 85, 105;
--cr-color-gray-700: 51, 65, 85;
--cr-color-gray-800: 30, 41, 59;
--cr-color-gray-900: 15, 23, 42;
--cr-color-yellow-50: 254, 252, 232;
--cr-color-yellow-100: 254, 249, 195;
--cr-color-yellow-200: 254, 240, 138;
--cr-color-yellow-300: 253, 224, 71;
--cr-color-yellow-400: 250, 204, 21;
--cr-color-yellow-500: 234, 179, 8;
--cr-color-yellow-600: 202, 138, 4;
--cr-color-yellow-700: 161, 98, 7;
--cr-color-yellow-800: 133, 77, 14;
--cr-color-yellow-900: 113, 63, 18;
--cr-color-blue-50: 239, 246, 255;
--cr-color-blue-100: 219, 234, 254;
--cr-color-blue-200: 191, 219, 254;
--cr-color-blue-300: 147, 197, 253;
--cr-color-blue-400: 96, 165, 250;
--cr-color-blue-500: 59, 130, 246;
--cr-color-blue-600: 37, 99, 235;
--cr-color-blue-700: 29, 78, 216;
--cr-color-blue-800: 30, 64, 175;
--cr-color-blue-900: 30, 58, 138;
--cr-color-red-50: 254, 242, 242;
--cr-color-red-100: 254, 226, 226;
--cr-color-red-200: 254, 202, 202;
--cr-color-red-300: 252, 165, 165;
--cr-color-red-400: 248, 113, 113;
--cr-color-red-500: 239, 68, 68;
--cr-color-red-600: 220, 38, 38;
--cr-color-red-700: 185, 28, 28;
--cr-color-red-800: 153, 27, 27;
--cr-color-red-900: 127, 29, 29;
--cr-color-green-50: 240, 253, 244;
--cr-color-green-100: 220, 252, 231;
--cr-color-green-200: 187, 247, 208;
--cr-color-green-300: 134, 239, 172;
--cr-color-green-400: 74, 222, 128;
--cr-color-green-500: 34, 197, 94;
--cr-color-green-600: 22, 163, 74;
--cr-color-green-700: 21, 128, 61;
--cr-color-green-800: 22, 101, 52;
--cr-color-green-900: 20, 83, 45;
--cr-color-emerald-50: 236, 253, 245;
--cr-color-emerald-100: 209, 250, 229;
--cr-color-emerald-200: 167, 243, 208;
--cr-color-emerald-300: 110, 231, 183;
--cr-color-emerald-400: 52, 211, 153;
--cr-color-emerald-500: 16, 185, 129;
--cr-color-emerald-600: 5, 150, 105;
--cr-color-emerald-700: 4, 120, 87;
--cr-color-emerald-800: 6, 95, 70;
--cr-color-emerald-900: 6, 78, 59;
--cr-color-teal-50: 240, 253, 250;
--cr-color-teal-100: 204, 251, 241;
--cr-color-teal-200: 153, 246, 228;
--cr-color-teal-300: 94, 234, 212;
--cr-color-teal-400: 45, 212, 191;
--cr-color-teal-500: 20, 184, 166;
--cr-color-teal-600: 13, 148, 136;
--cr-color-teal-700: 15, 118, 110;
--cr-color-teal-800: 17, 94, 89;
--cr-color-teal-900: 19, 78, 74;
--cr-color-purple-50: 250, 245, 255;
--cr-color-purple-100: 243, 232, 255;
--cr-color-purple-200: 233, 213, 255;
--cr-color-purple-300: 216, 180, 254;
--cr-color-purple-400: 192, 132, 252;
--cr-color-purple-500: 168, 85, 247;
--cr-color-purple-600: 147, 51, 234;
--cr-color-purple-700: 126, 34, 206;
--cr-color-purple-800: 107, 33, 168;
--cr-color-purple-900: 88, 28, 135;
--cr-color-amber-50: 255, 251, 235;
--cr-color-amber-100: 254, 243, 199;
--cr-color-amber-200: 253, 230, 138;
--cr-color-amber-300: 252, 211, 77;
--cr-color-amber-400: 251, 191, 36;
--cr-color-amber-500: 245, 158, 11;
--cr-color-amber-600: 217, 119, 6;
--cr-color-amber-700: 180, 83, 9;
--cr-color-amber-800: 146, 64, 14;
--cr-color-amber-900: 120, 53, 15;
--cr-color-white: 255, 255, 255;
--cr-color-black: 4, 4, 5;
--cr-color-highlight: 56, 189, 248;
}
Payments Onboarding
This component displays the current onboarding status by default and provides an option to start the onboarding process if it is not completed yet. It is usually used on the dashboard of the application after the user is logged in.
Preview
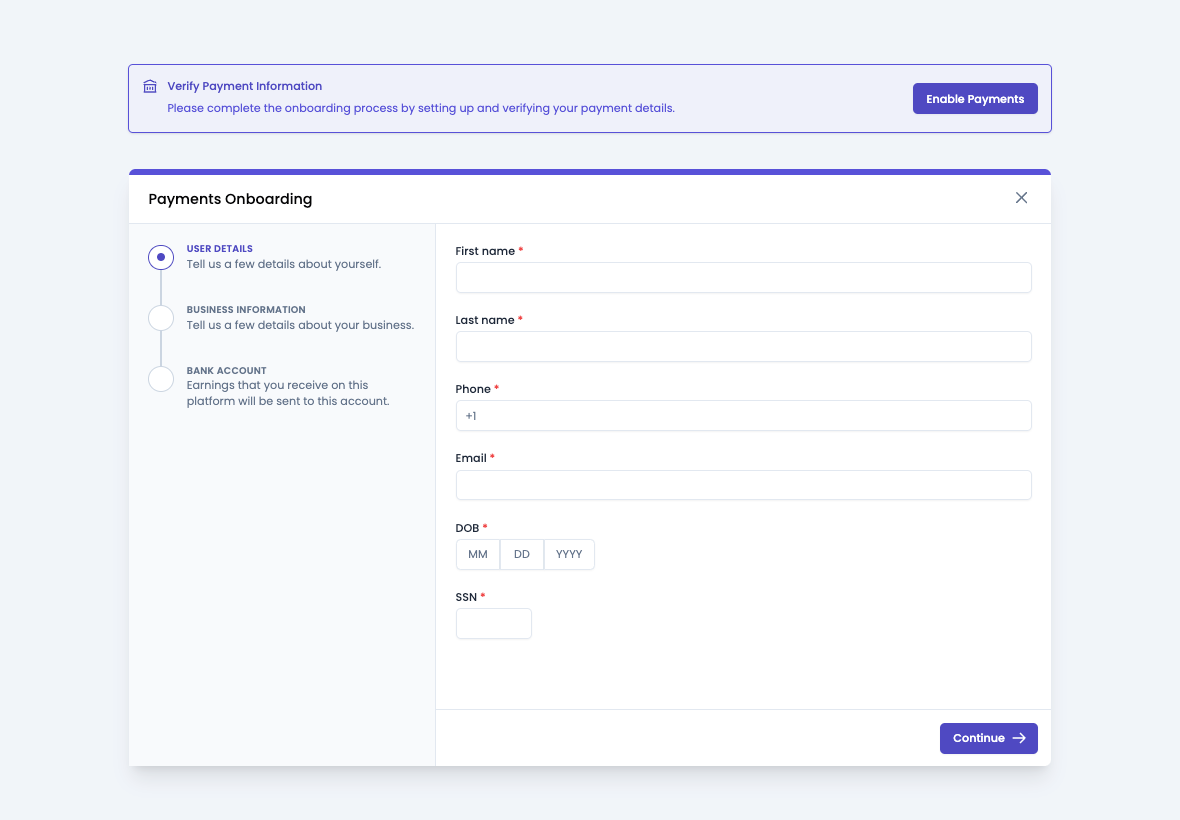
Usage
Import the BusinessPaymentStatus component and use it in your application as shown below:
PaymentStatus.tsx
import { BusinessPaymentStatus } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<BusinessPaymentStatus />
</>
);
Payment Element
This component renders a payment form for paying a single invoice. It supports all the payment methods that are configured in your tenant settings.
Preview
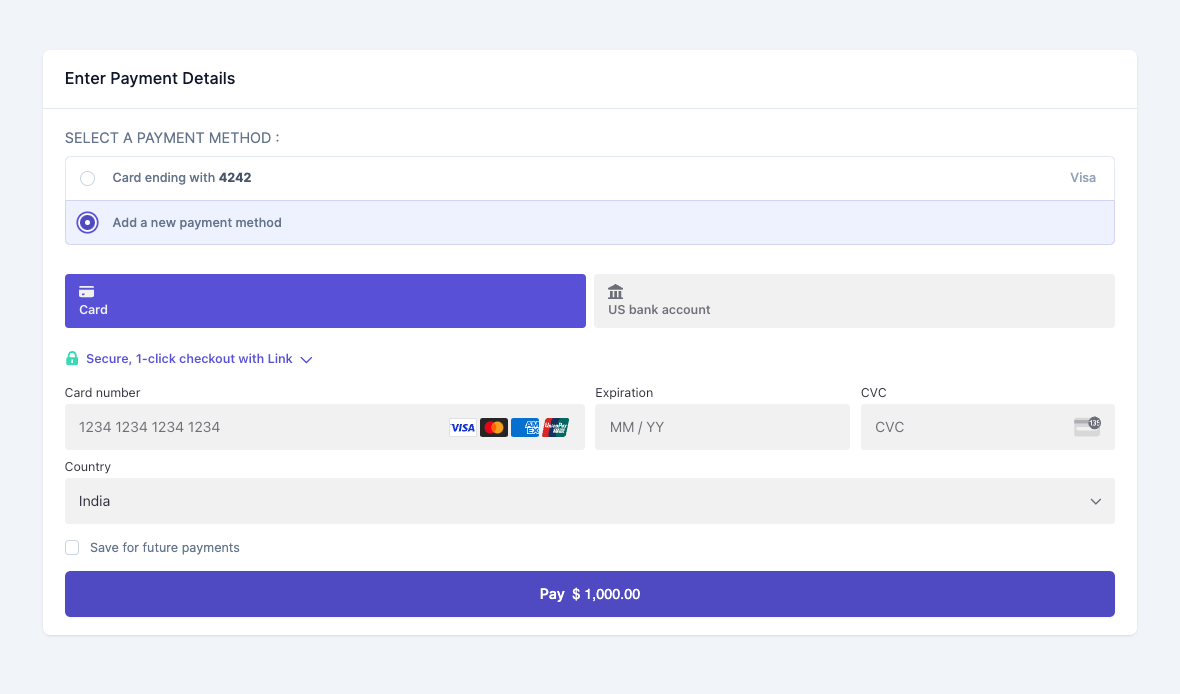
Usage
Import the PaymentElement component and use it in your application as shown below:
PaymentElement.tsx
import { PaymentElement } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<PaymentElement
invoiceId="9c160840-14ec-4a47-9eb4-5fe79237e0ee"
onSuccess={onSuccess}
onError={onError}
/>
</>
)
Props
- Name
invoiceId
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the invoice to be paid.
Events
- Name
onSuccess
- Type
- Field Type
- Description
Triggered when payment is succeeded.
- Name
onError
- Type
- Field Type
- Description
Triggered when payment has error.
Customer Table
This component displays a table of all customers. The component shows each customer's name, email and amount due as well as other required details.
Preview
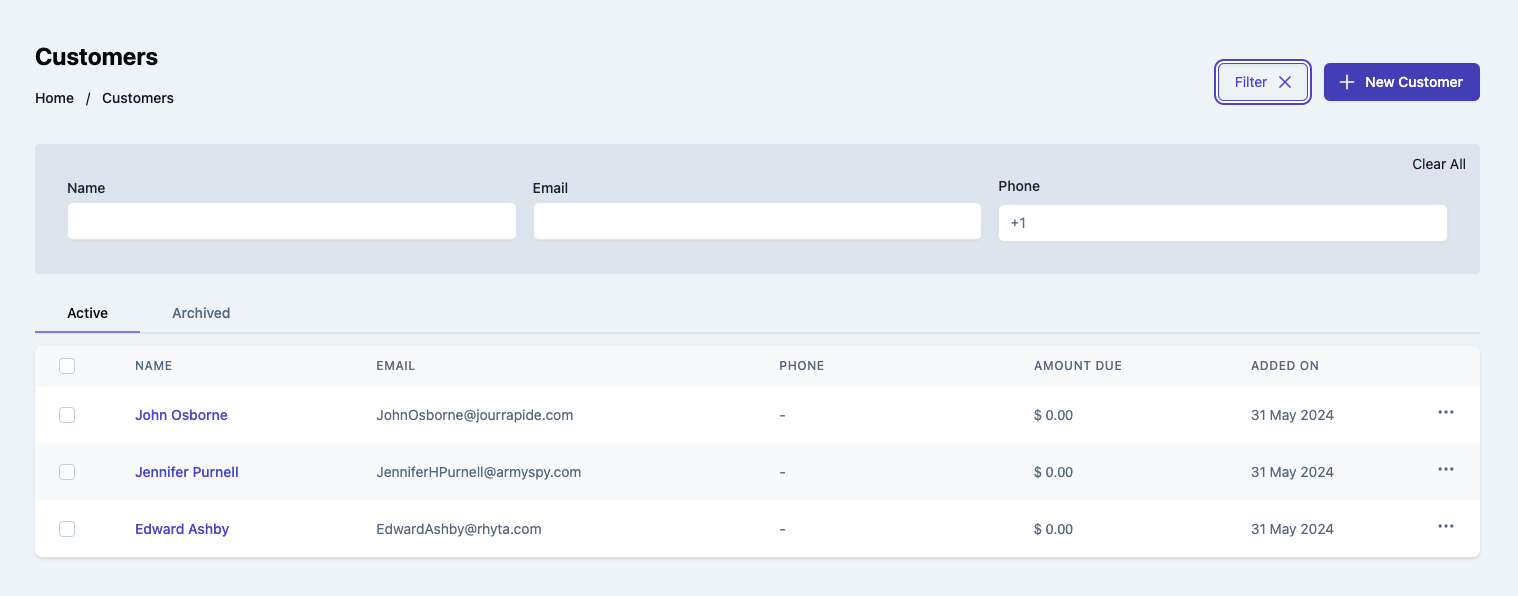
Usage
Renders a table of customers. Use the CustomerTable component in your application as shown below:
Customers.tsx
import { CustomerTable } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<CustomerTable
showFilters
showCreateButton
showViewOption
onRowClick={onRowClick}
onViewOptionClick={onViewOptionClick}
/>
</>
);
Props
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide customer create button. default value is
true
.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide customer table title. default value is
true
.
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button. default value is
true
.
- Name
type
- Type
- string
- Field Type
optional
- Description
This prop accepts 'both' | 'active' | 'archived' for displaying tabs. default value is
both
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
name
,email
,phone
,archived
, key and its value for applying filter.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['checkbox', 'name', 'email', 'phone', 'due_amount', 'created_at', 'actions']
Example:
If you pass['name', 'email', 'phone', 'due_amount',]
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<CustomerTable columns="[{ key: 'email', label: 'Customer Email', thClass: 'text-left', tdClass: 'text-left', sortable: true }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<CustomerTable columns="[ 'name', { key: 'email', label: 'Email Address', sortable: false } ] />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a customer table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when customer create button clicked.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when customer view option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in customer table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in customer table component.
<CustomerTable>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>Customers</h1>
<button type="button" className="mr-2">
New Customer
</button>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" onClick={() => {}} />
<BreadcrumbItem title="Customers" active />
</Breadcrumb>
)
}}
</CustomerTable>
Customer Details
This component renders UI to view details of a single customer and allows performing various actions on the customer.
Preview
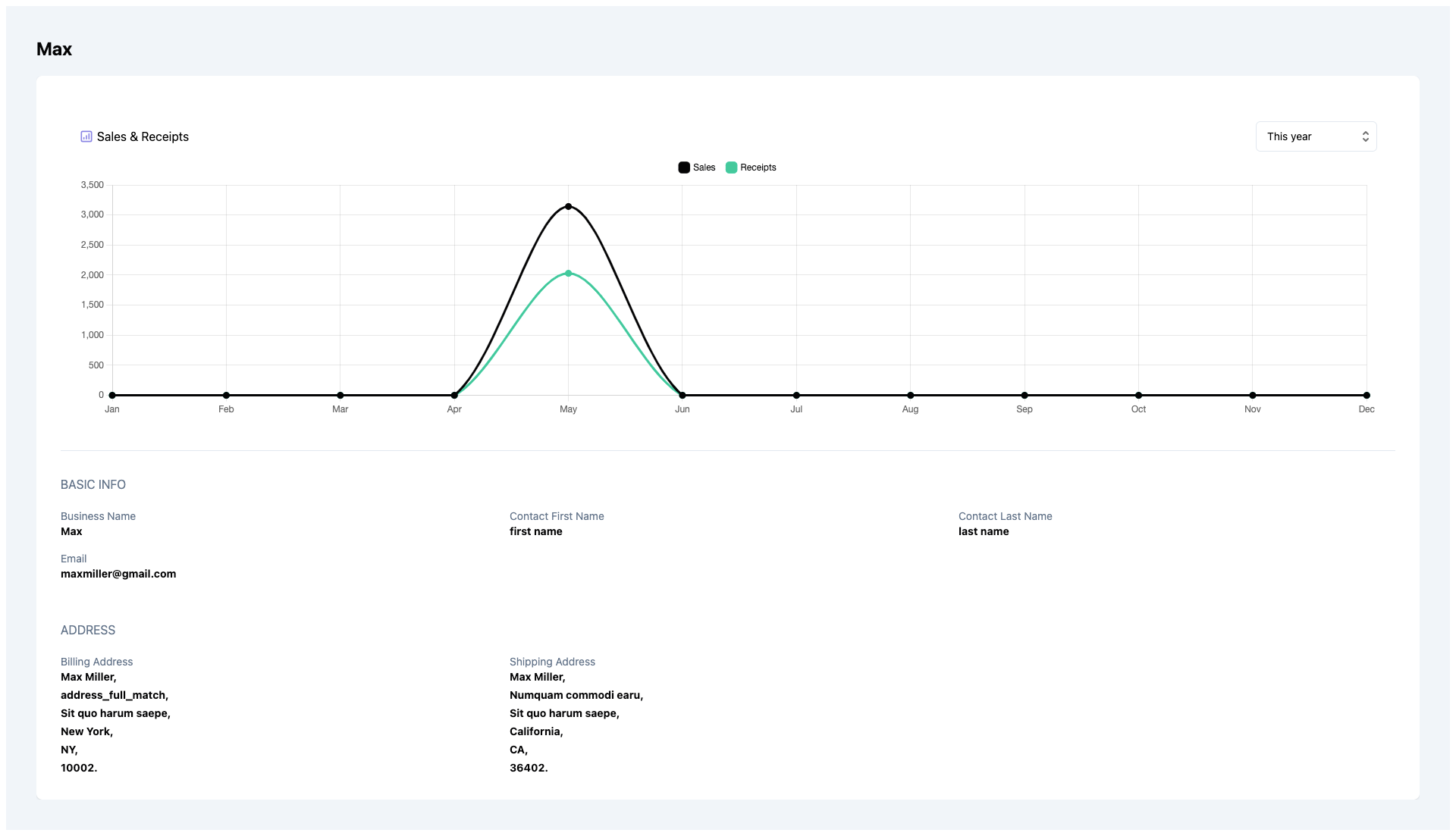
Usage
Use the CustomerDetails component to view details of an existing customer.
Customer.tsx
import { CustomerDetails } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<CustomerDetails id="CUSTOMER_ID" />
</>
);
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the customer details to be displayed.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in customer details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in customer details component.
<CustomerDetails id="CUSTOMER_ID">
{{
header: (customer) => (
<div className="flex items-center justify-between">
<h1>Customer details {customer.name}</h1>
<button type="button" className="mr-2">
Go Back
</button>
</div>
),
breadcrumb: (customer) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Customers" />
<BreadcrumbItem title={customer?.name} active />
</Breadcrumb>
)
}}
</CustomerDetails>
Customer Create
This component serves as an interface for users to input and submit information essential for creating a new customer. It handles the UI elements, data validation, and submission of the form.
Preview
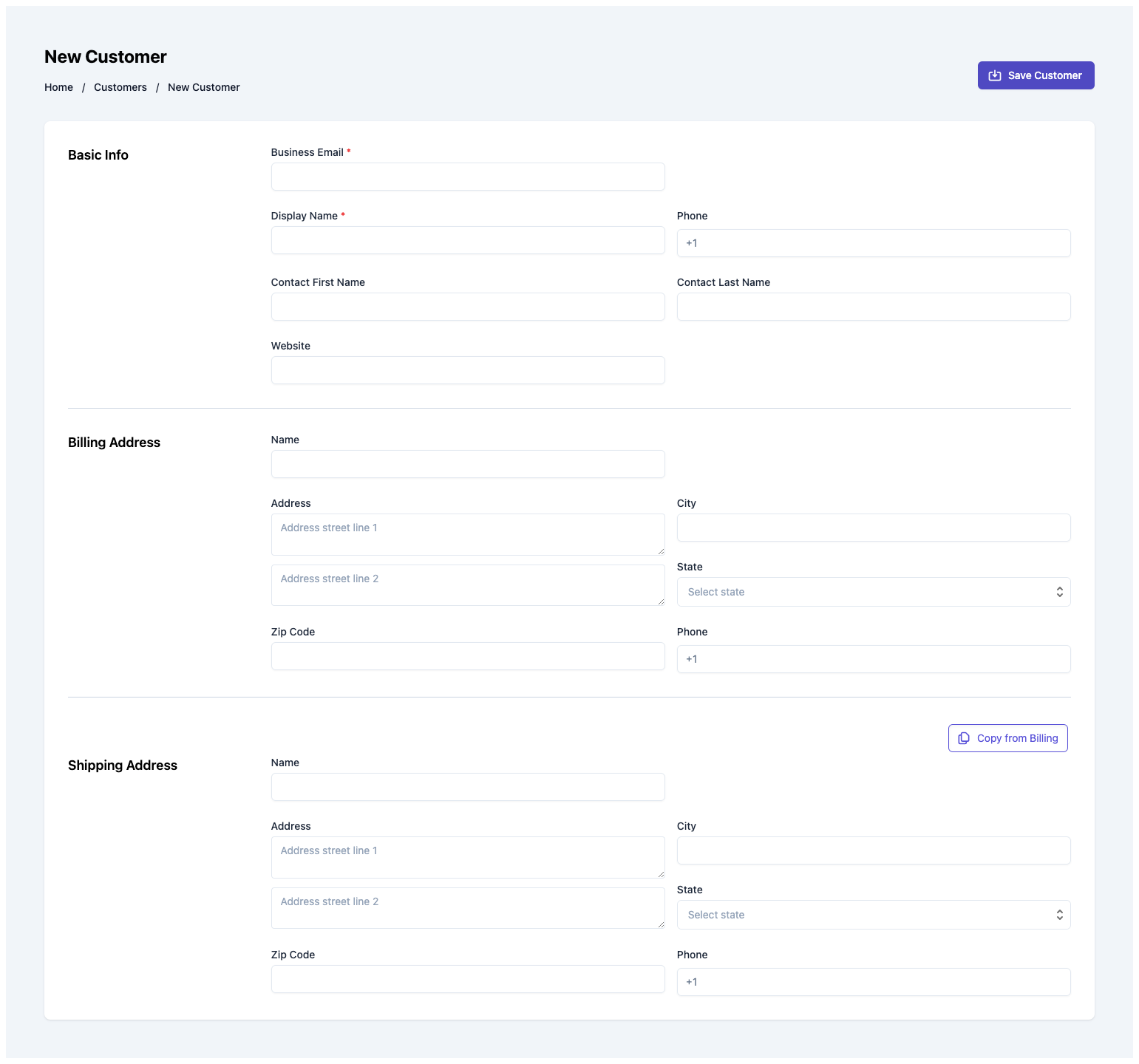
Usage
Renders a form for creating a new customer. Use the CustomerCreate component in your application as shown below:
CustomerCreate.tsx
import { CustomerCreate } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<CustomerCreate showDetailOnSuccess={true} onCreate={onCreate} />
</>
);
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide customer details component after customer created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- function
- Field Type
- Description
Triggered when a customer is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in customer create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in customer create component.
<CustomerCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Customer</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Customers" />
<BreadcrumbItem title="New Customer" active />
</Breadcrumb>
),
}}
</CustomerCreate>
Vendor Table
This component displays a table of all vendors. The component shows each vendor's name, email and phone as well as other required details.
Preview
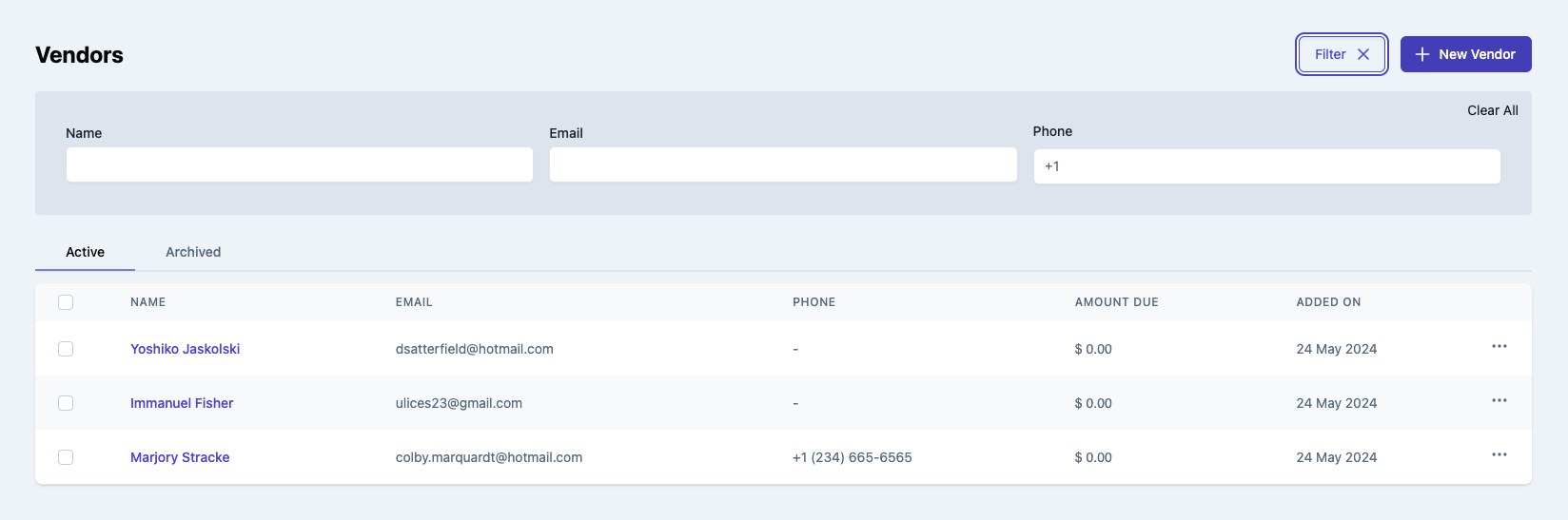
Usage
Renders a table of vendors. Use the VendorTable component in your application as shown below:
Vendors.tsx
import { VendorTable } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<VendorTable
showFilters
showCreateButton
showViewOption
onRowClick={onRowClick}
onViewOptionClick={onViewOptionClick}
/>
</>
);
Props
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide vendor create button. default value is
true
.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide vendor table title. default value is
true
.
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button. default value is
true
.
- Name
type
- Type
- string
- Field Type
optional
- Description
This prop accepts 'both' | 'active' | 'archived' for displaying tabs. default value is
both
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
name
,email
,phone
,archived
, key and its value for applying filter.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['checkbox', 'name', 'email', 'phone', 'due_amount', 'created_at', 'actions']
Example:
If you pass['name', 'email', 'phone', 'due_amount',]
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<VendorTable columns="[{ key: 'email', label: 'Vendor Email', thClass: 'text-left', tdClass: 'text-left', sortable: true }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<VendorTable columns="[ 'name', { key: 'email', label: 'Email Address', sortable: false } ] />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a vendor table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when vendor create button clicked.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when vendor view option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in vendor table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in vendor table component.
<VendorTable>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>Vendors</h1>
<button type="button" className="mr-2">
New Vendor
</button>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" onClick={() => {}} />
<BreadcrumbItem title="Vendors" active />
</Breadcrumb>
)
}}
</VendorTable>
Vendor Details
This component renders the interface for displaying an existing vendor.
Preview
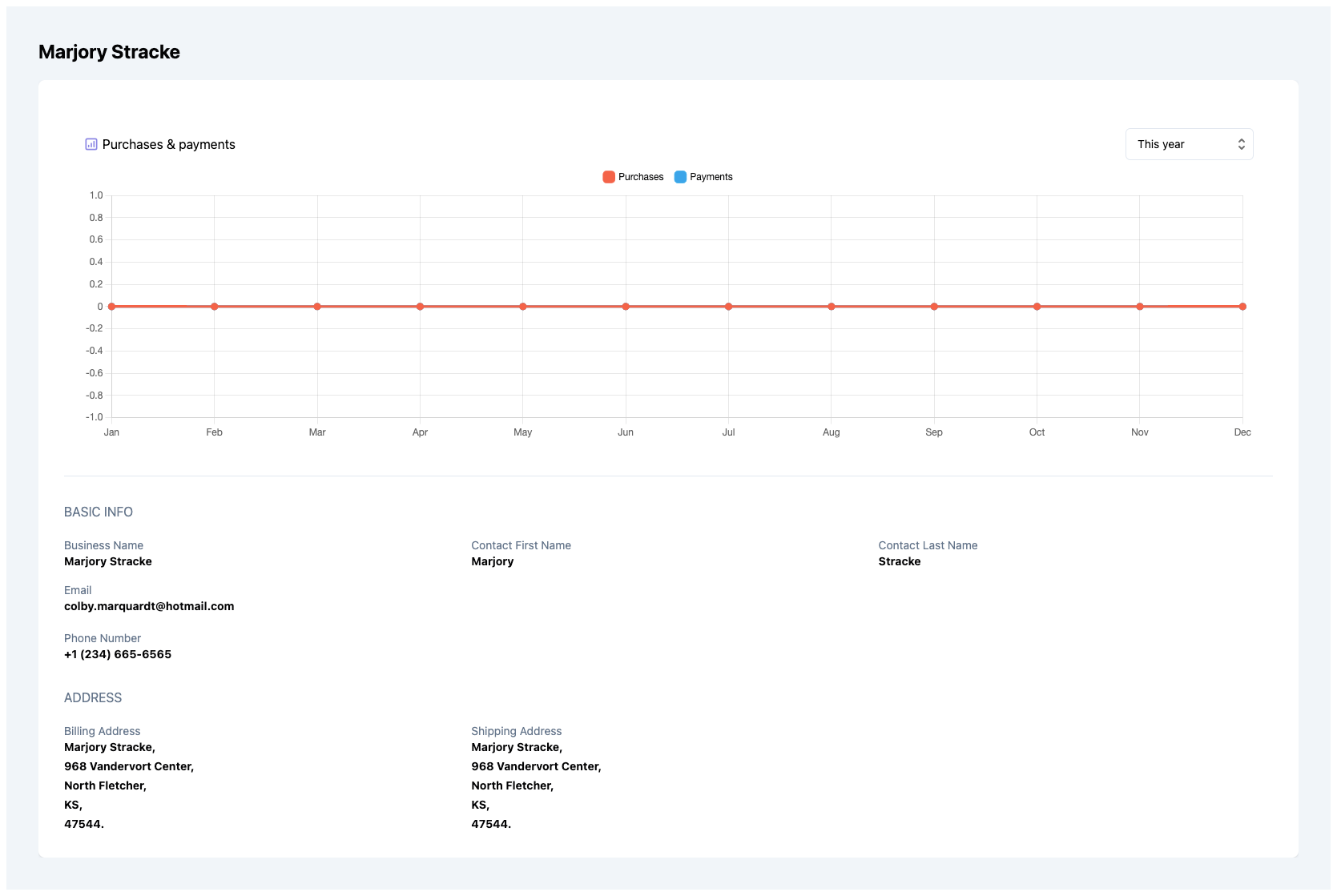
Usage
Use the VendorDetails component to view details of an existing vendor.
Vendor.tsx
import { VendorDetails } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<VendorDetails id="VENDOR_ID" />
</>
);
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the vendor details to be displayed.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in vendor details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in vendor details component.
<VendorDetails id="VENDOR_ID">
{{
header: (vendor) => (
<div className="flex items-center justify-between">
<h1>Vendor details {vendor.name}</h1>
<button type="button" className="mr-2">
Go Back
</button>
</div>
),
breadcrumb: (vendor) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Vendors" />
<BreadcrumbItem title={vendor?.name} active />
</Breadcrumb>
)
}}
</VendorDetails>
Vendor Create
This component serves as an interface for users to input and submit information essential for generating a new vendor. It handles the UI elements & data validation to create vendor within your application.
Preview
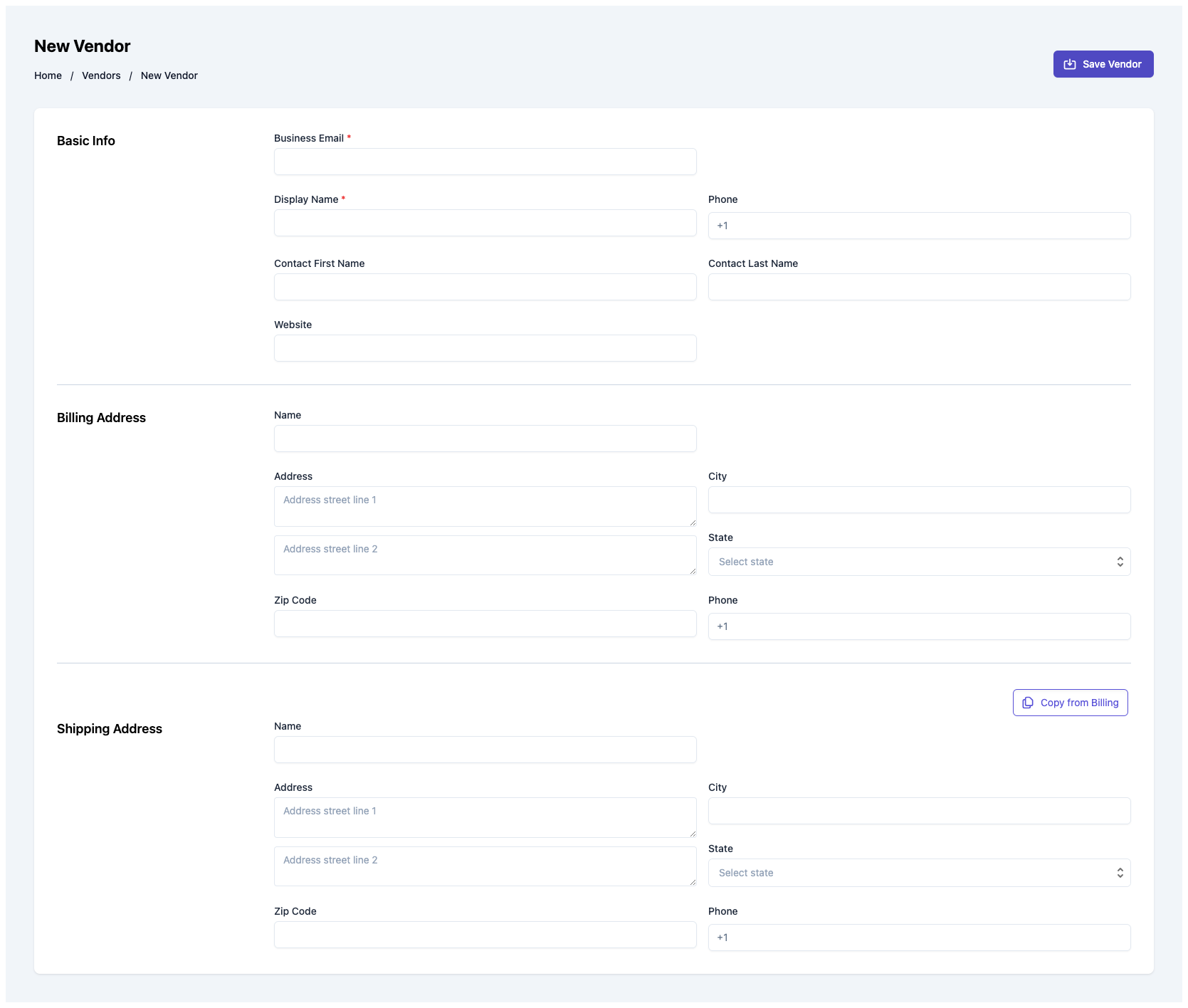
Usage
Renders a form for creating a new vendor. Use the VendorCreate component in your application as shown below:
VendorCreate.tsx
import { VendorCreate } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<VendorCreate showDetailOnSuccess={true} onCreate={onCreate} />
</>
);
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide vendor details component after vendor created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- function
- Field Type
- Description
Triggered when a vendor is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in customer create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in vendor create component.
<VendorCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Vendor</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Vendors" />
<BreadcrumbItem title="New Vendor" active />
</Breadcrumb>
),
}}
</VendorCreate>
Item Table
This component displays a table of all items. The component shows each item's name, unit name and created at as well as other required details.
Preview
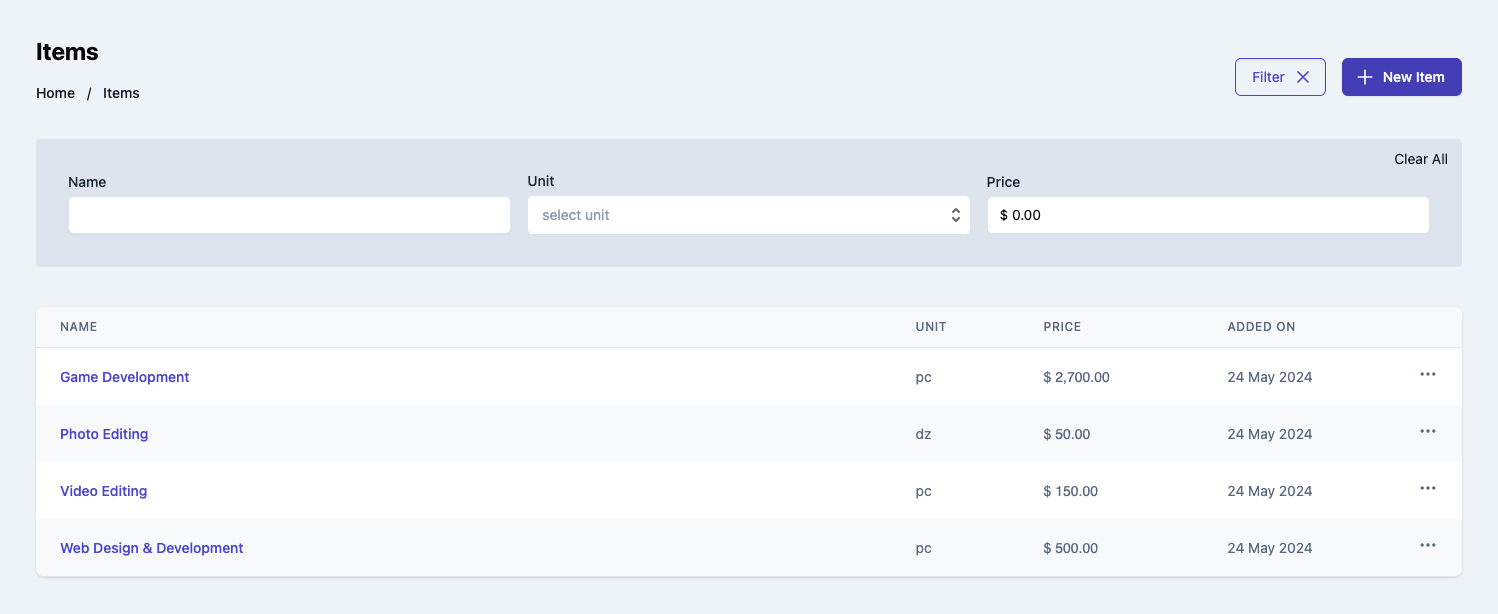
Usage
Renders a table of items. Use the ItemTable component in your application as shown below:
ItemTable.tsx
import { ItemTable } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<ItemTable
showCreateButton
showEditOption
showViewOption
showDeleteOption
onRowClick={onRowClick}
onEditOptionClick={onEditOptionClick}
onViewOptionClick={onViewOptionClick}
onCreateButtonClick={onCreateButtonClick}
/>
</>
)
Props
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item create button, default value is
true
.
- Name
showViewOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item view button, default value is
true
.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item edit button, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item delete option, default value is
true
.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item table title, default value is
true
.
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button, default value is
true
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
name
,unit
,price
key and its value for applying filter.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['name', 'unit_name', 'price', 'created_at', 'actions']
Example:
If you pass['unit_name', 'price', 'created_at']
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<ItemTable columns="[{ key: 'name', label: 'Item name', thClass: 'table-cell', tdClass: 'table-cell', sortable: true, }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<ItemTable columns="[ 'name', { key: 'price', label: 'Price', sortable: false } ]" />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a item table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when item create button clicked.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when item view option clicked from options menu.
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when item edit option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in items table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in items table component.
<ItemTable>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>Items</h1>
<button type="button" className="mr-2">
New Item
</button>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" onClick={() => {}} />
<BreadcrumbItem title="Items" active />
</Breadcrumb>
)
}}
</ItemTable>
Item Details
This component renders the interface for displaying an existing item. It also allows performing various actions on the item such as editing and deleting it.
Preview
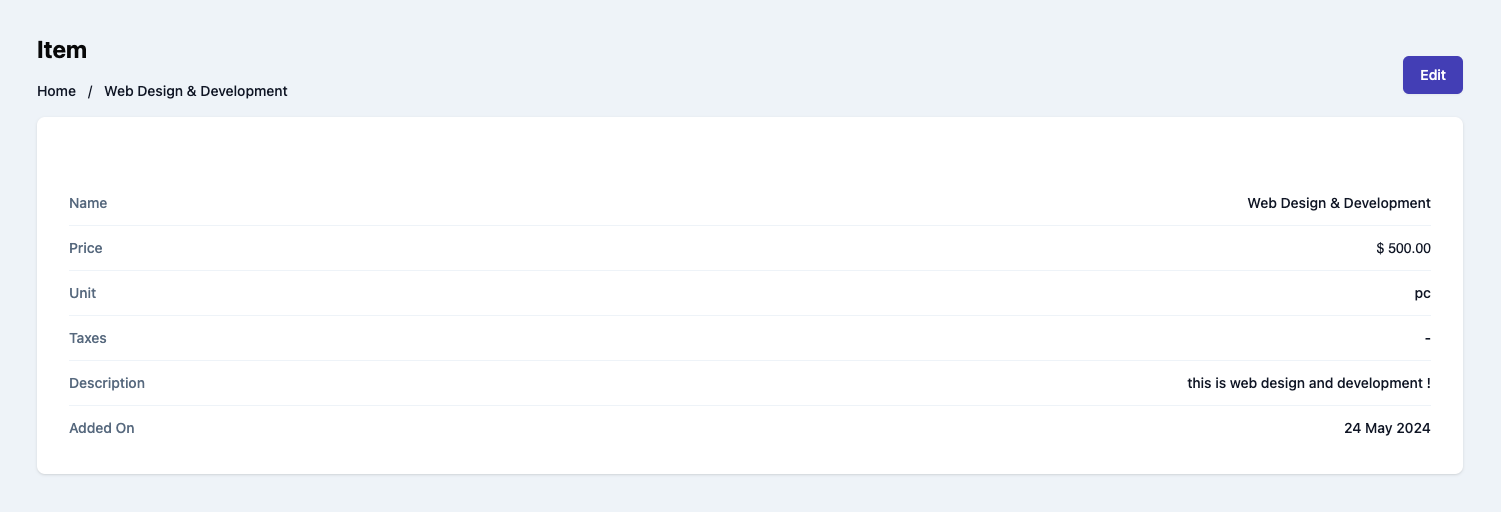
Usage
Use the ItemDetails component to view details of an existing item.
ItemDetails.tsx
import { ItemDetails } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<ItemDetails id="ITEM_ID" showEditOption onEditOptionClick={onItemEdit} />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the item details to be displayed.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide edit option, default value is
true
.
Events
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when item edit button clicked.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in item details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in item details component.
<ItemDetails id="ITEM_ID">
{{
header: (item) => (
<div className="flex justify-between">
<h1 className="text-2xl">Items</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
Edit
</button>
</div>
</div>
),
breadcrumb: (item) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Items" />
<BreadcrumbItem title={item?.name} active />
</Breadcrumb>
),
}}
</ItemDetails>
Item Create
This component serves as an interface for users to input and submit information essential for generating a new item. It handles the UI elements & data validation to create items within your application.
Preview
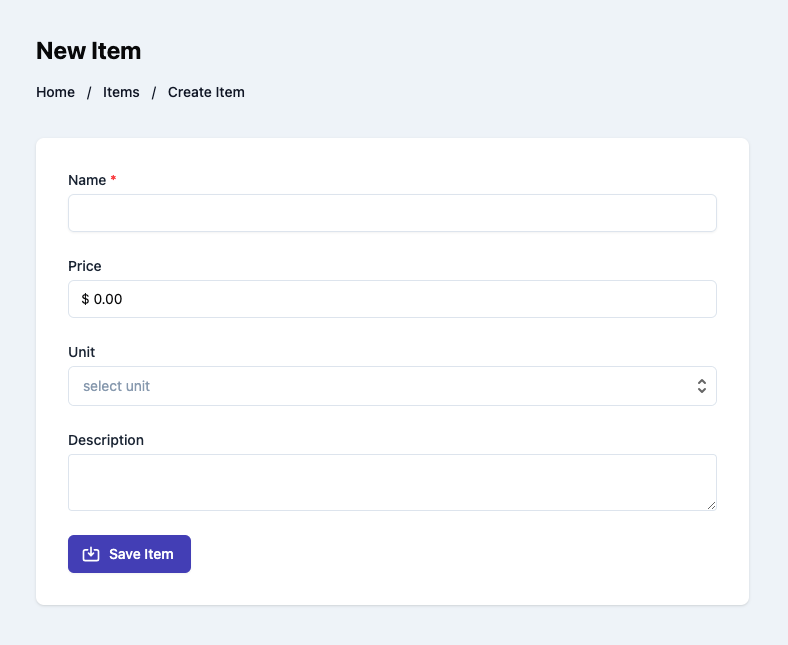
Usage
Renders a form for creating a new item. Use the ItemCreate component in your application as shown below:
ItemCreate.tsx
import { ItemCreate } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<ItemCreate showDetailOnSuccess={true} onCreate={onItemCreate} />
</>
)
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item details component after item created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- function
- Field Type
- Description
Triggered when a item is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in item create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in item create component.
<ItemCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Item</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Items" />
<BreadcrumbItem title="New Item" active />
</Breadcrumb>
),
}}
</ItemCreate>
Item Edit
This component renders a form for editing a pre-existing item. It encompasses functionalities tailored to allow users to update specific details of an item.
Preview
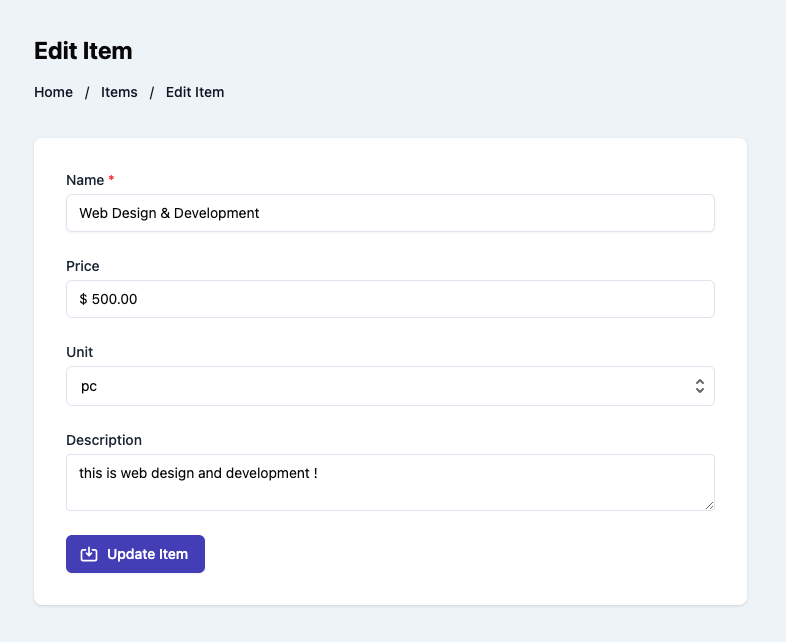
Usage
Renders a form for editing an existing item. Use the ItemEdit component in your application as shown below:
ItemEdit.tsx
import { ItemEdit } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<ItemEdit id="ITEM_ID" showDetailOnSuccess={false} onUpdate={onItemEdit} />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the item.
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide item details component after item updated successfully, default value is
true
.
Events
- Name
onUpdate
- Type
- Field Type
- Description
Triggered when the item is updated successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in item edit component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in item edit component.
<ItemEdit id="ITEM_ID">
{{
header: (item) => (
<div className="flex items-center justify-between">
<h1>Edit Item</h1>
</div>
),
breadcrumb: (item) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Items" />
<BreadcrumbItem title="Edit Item" active />
</Breadcrumb>
),
}}
</ItemEdit>
Estimate Table
This component displays all estimates created by a business. The component shows each estimate's amount, status, due date, overdue date, and the counterpart to which they were sent.
Preview
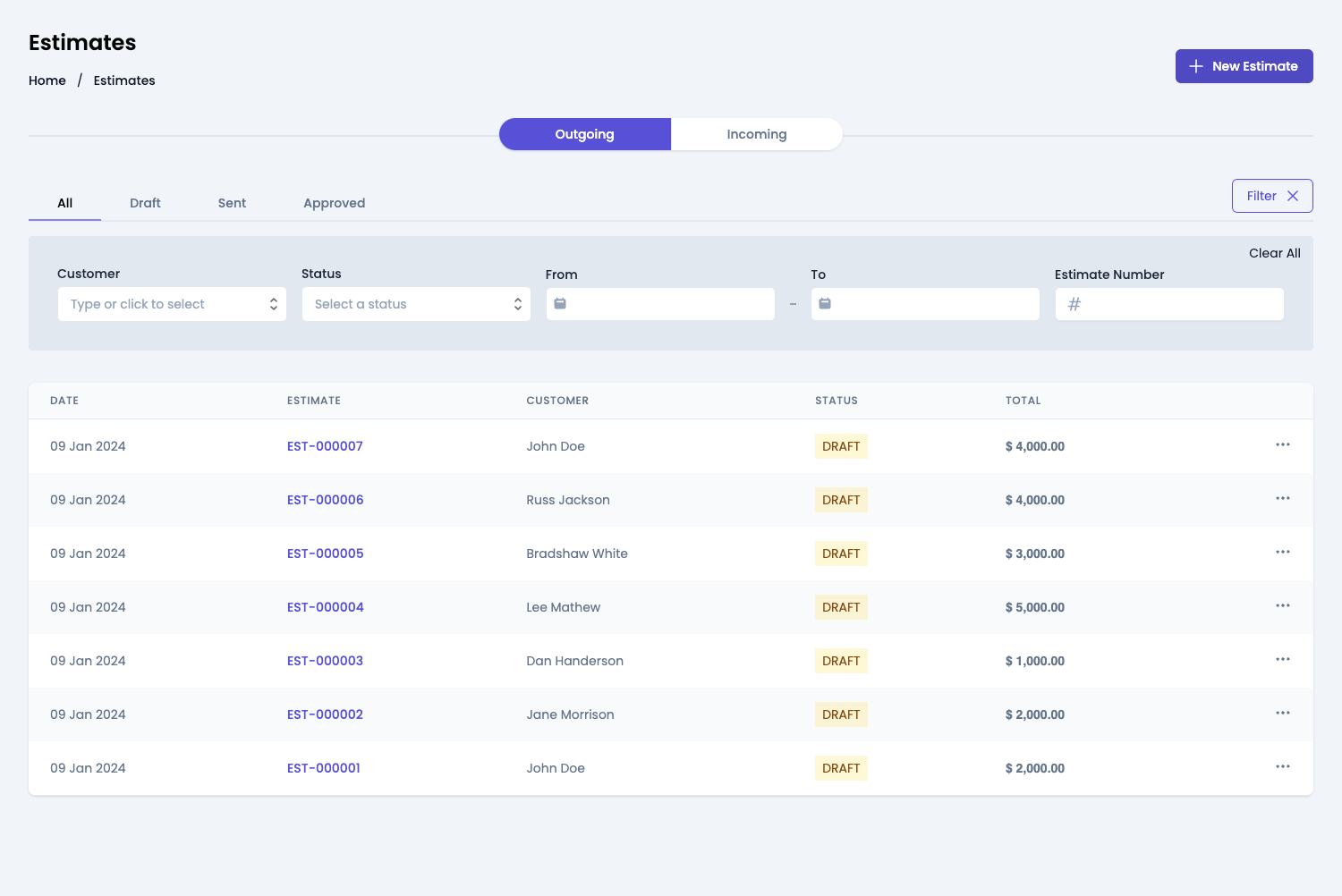
Usage
Import the EstimateTable component and use it in your application as shown below:
Estimates.tsx
import { EstimateTable } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateTable
showCreateButton
showFilters
showTabs
showEditOption
showSendEstimateOption
showDeleteOption={false}
showApproveEstimateOption={false}
showRejectEstimateOption={false}
showFinalizeEstimateOption={false}
showVoidInvoiceOption={false}
onCreateButtonClick={onEstimateCreate}
onEditOptionClick={onEstimateEdit}
onViewOptionClick={onEstimateView}
/>
</>
)
Props
- Name
type
- Type
- string
- Field Type
optional
- Description
This is prop accepts type with value
incoming
,outgoing
andboth
, default value isboth
.
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button, default value is
true
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
customer_id
,status
,from_date
,to_date
,estimate_number
key and its value for applying filter.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate table title, default value is
true
.
- Name
showTabs
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate status tabs, default value is
true
.
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate create button, default value is
true
.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate edit option in table, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate delete option in table, default value is
true
.
- Name
showSendEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate send option in table, default value is
true
.
- Name
showApproveEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate approve option in table, default value is
true
.
- Name
showRejectEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate reject option in table, default value is
true
.
- Name
showFinalizeEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate finalize option in table, default value is
true
.
- Name
showVoidEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate void option in table, default value is
true
.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['checkbox', 'estimate_date', 'estimate_number', 'business_name', 'status', 'total', 'convert', 'actions']
Example:
If you pass['estimate_date', 'estimate_number', 'business_name', 'status']
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<EstimateTable columns="[{ key: 'estimate_number', label: 'Estimate Number', thClass: 'text-left', tdClass: 'text-left', sortable: true, }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<EstimateTable columns="[ 'estimate_number', { key: 'status', label: 'Status', sortable: false } ] />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a estimate table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when estimate create button clicked.
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when estimate edit option clicked from options menu.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when estimate view option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in estimate table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in estimate table component.
<EstimateTable>
{{
header: () => (
<div className="flex justify-between">
<h1 className="text-2xl">Estimates</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
New Estimate
</button>
</div>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Estimates" active />
</Breadcrumb>
),
}}
</EstimateTable>
Estimate Details
This component renders the interface for displaying an existing estimate. It also allows performing various actions on the estimate such as editing, deleting, and sending it.
Preview
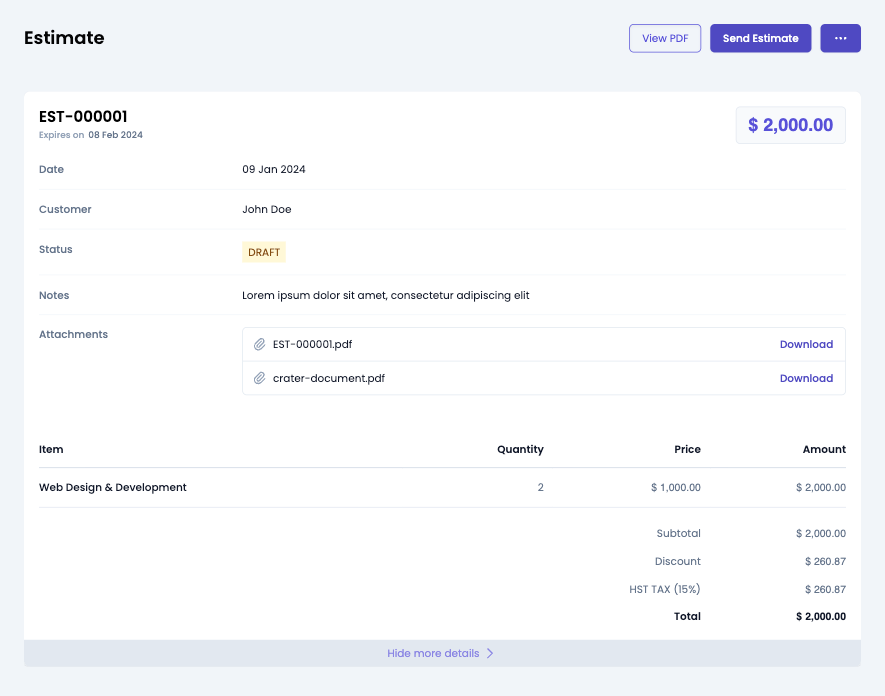
Usage
Use the EstimateDetails component to view details of an existing estimate.
EstimateDetails.tsx
import { EstimateDetails } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateDetails
id="ESTIAMTE_ID"
showEditOption
showDeleteOption
showSendEstimateOption
showApproveEstimateOption
showFinalizeInvoiceOption
showRejectEstimateOption={false}
showVoidInvoiceOption={false}
onEditOptionClick={onEstimateEdit}
/>
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the estimate details to be displayed.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide edit option, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide delete option, default value is
true
.
- Name
showSendEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide send estimate option, default value is
true
.
- Name
showApproveEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide approve estimate option, default value is
true
.
- Name
showRejectEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide reject estimate option, default value is
true
.
- Name
showVoidEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide void estimate option, default value is
true
.
- Name
showFinalizeEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide finalize estimate button, default value is
true
.
Events
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when invoice edit option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in estimate details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in estimate details component.
<EstimateDetails id="ESTIMATE_ID">
{{
header: (estimate) => {
return (
<div className="flex justify-between">
<h1 className="text-2xl">Estimate details</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
Edit
</button>
</div>
</div>
)
},
breadcrumb: (estimate) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Estimates" />
<BreadcrumbItem title={estimate?.estimate_number} active />
</Breadcrumb>
),
}}
</EstimateDetails>
Estimate Create
This component serves as an interface for users to input and submit information essential for generating a new estimate. It handles the UI elements & data validation to create estimates within your application.
Preview
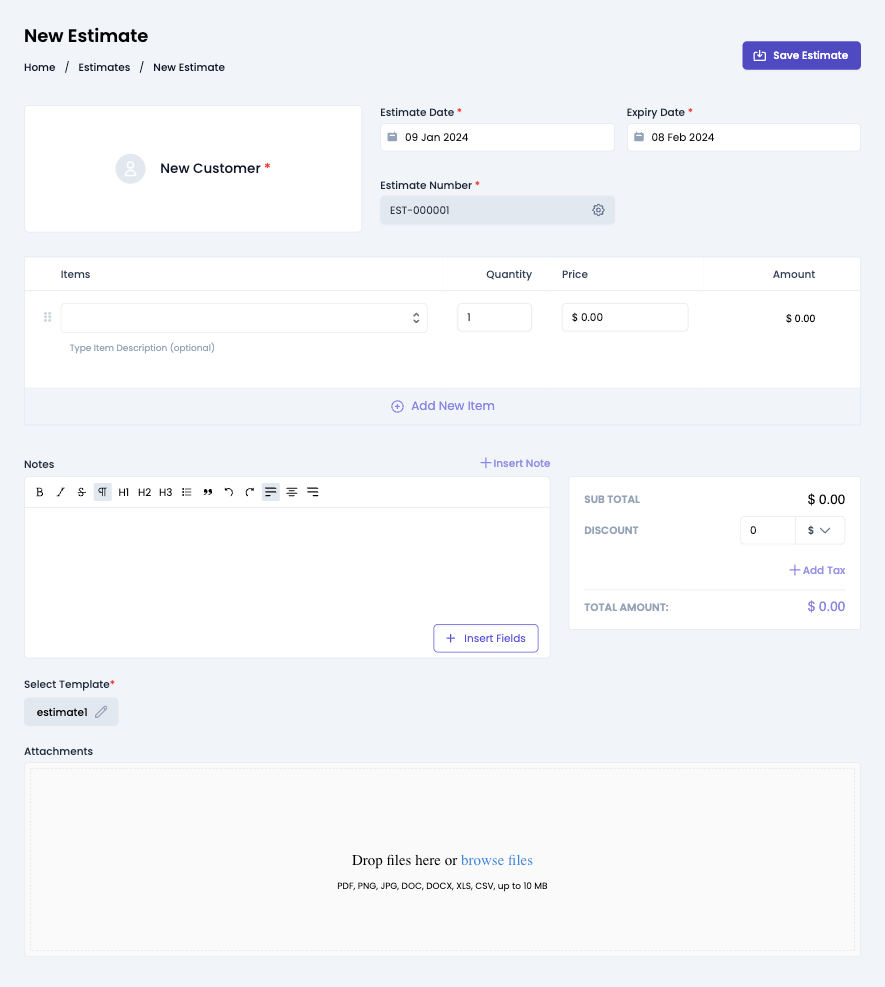
Usage
Import the EstimateCreate component and use it in your application as shown below:
EstimateCreate.tsx
import { EstimateCreate } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateCreate showDetailOnSuccess={false} onCreate={onEstimatecreate} />
</>
)
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate details component after estimate created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- Field Type
- Description
Triggered when an estimate is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in estimate create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in estimate create component.
<EstimateCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Estimate</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Estimates" />
<BreadcrumbItem title="New Estimate" active />
</Breadcrumb>
),
}}
</EstimateCreate>
Estimate Edit
This component renders a form for editing a pre-existing estimate. It encompasses functionalities tailored to allow users to update specific details of an estimate.
Preview
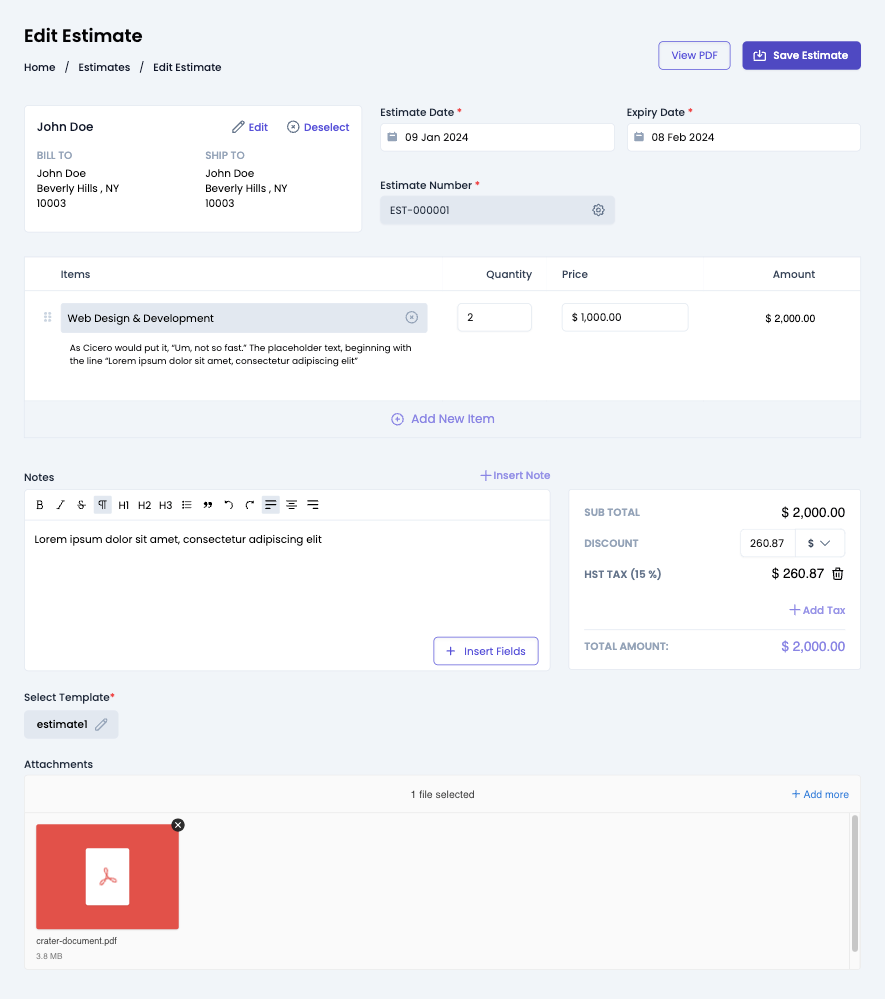
Usage
Import the EstimateEdit component and use it in your application as shown below:
EstimateEdit.tsx
import { EstimateEdit } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateEdit id="ESTIMATE_ID" showDetailOnSuccess={false} onUpdate={onEstimateEdit} />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the estimate.
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide estimate details component after estimate updated successfully.
Events
- Name
onUpdate
- Type
- Field Type
- Description
Triggered when the estimate is updated successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in estimate edit component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in estimate edit component.
<EstimateEdit id="ESTIMATE_ID">
{{
header: (estimate) => (
<div className="flex items-center justify-between">
<h1>Edit Estimate</h1>
</div>
),
breadcrumb: (estimate) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Estimates" />
<BreadcrumbItem title="Edit Estimate" active />
</Breadcrumb>
),
}}
</EstimateEdit>
Invoice Table
This component displays all invoices created by a business. The component shows each invoice's amount, status, due date, overdue date, and the counterpart to which they were sent.
Preview
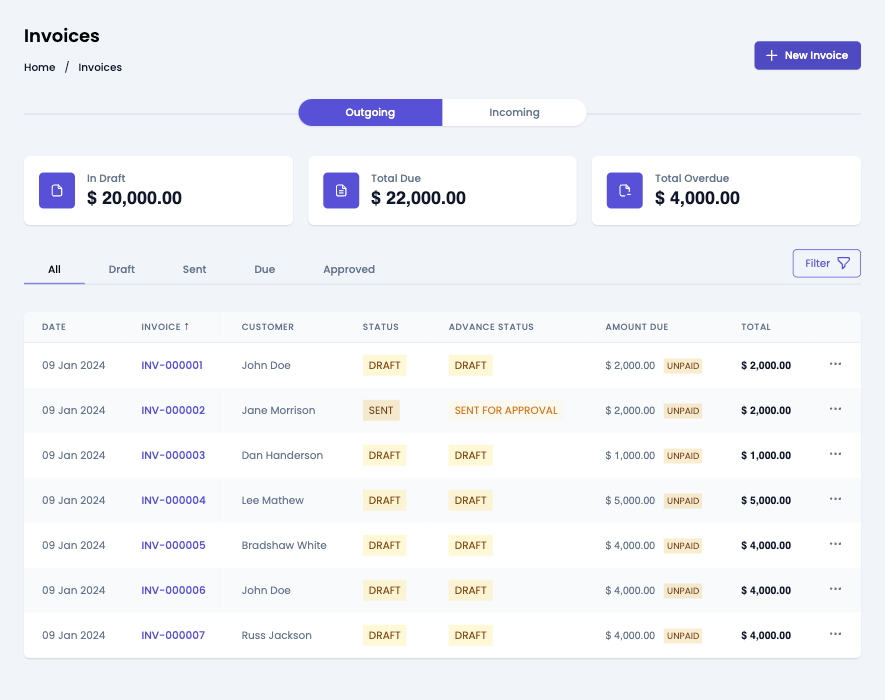
Usage
Renders a table of invoices. Use in the InvoiceTable component in your application as shown:
Invoices.tsx
import { InvoiceTable } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<InvoiceTable
showStats
showFilters
showTabs
showCreateButton
showEditOption
showDeleteOption={false}
showSendInvoiceOption={false}
showCloneInvoiceOption={false}
showVoidInvoiceOption={false}
onCreateButtonClick={onCreateInvoice}
onEditOptionClick={onEditInvoice}
onViewOptionClick={onViewInvoice}
/>
</>
)
Props
- Name
showStats
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide stats section, default value is
true
.
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button, default value is
true
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
customer_id
,status
,paid_status
,from_date
,to_date
,invoice_number
,tab_status
key and its value for applying filter.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice table title, default value is
true
.
- Name
showTabs
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice status tabs, default value is
true
.
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice create button, default value is
true
.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice edit option in table, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice delete option in table, default value is
true
.
- Name
showSendInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice send option in table, default value is
true
.
- Name
showCloneInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice clone option in table, default value is
true
.
- Name
showApproveInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice approve option in table, default value is
true
.
- Name
showFinalizeInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice finalize option in table, default value is
true
.
- Name
showVoidEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice void option in table, default value is
true
.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['checkbox', 'invoice_date', 'invoice_number', 'business_name', 'status', 'loan_status', 'due_amount', 'total', 'advance', 'actions']
Example:
If you pass['invoice_number', 'customer', 'total', 'due_date', 'status']
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<InvoiceTable columns="[{ key: 'invoice_number', label: 'Invoice Number', thClass: 'text-left', tdClass: 'text-left', sortable: true, }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<InvoiceTable columns="[ 'invoice_number', { key: 'status', label: 'Status', sortable: false } ] />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a invoice table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when invoice create button clicked.
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when invoice edit option clicked from options menu.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when invoice view option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in invoice table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in invoice table component.
<InvoiceTable>
{{
header: () => (
<div className="flex justify-between">
<h1 className="text-2xl">Invoices</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
New Invoice
</button>
</div>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Invoices" active />
</Breadcrumb>
),
}}
</InvoiceTable>
Invoice Details
This component renders the interface for displaying an existing invoice. It also allows performing various actions on the invoice such as editing, deleting, and sending it.
Preview
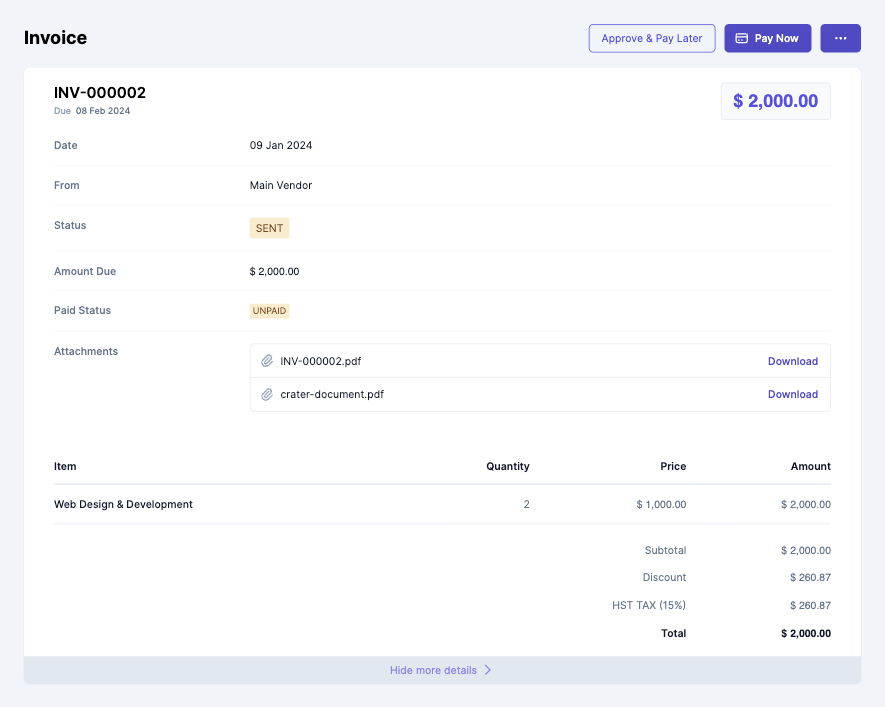
Usage
To view details of an existing invoice, use the InvoiceDetails component with the id prop as shown:
InvoiceDetails.tsx
import { InvoiceDetails } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<InvoiceDetails
id="INVOICE_ID"
showEditOption
showSendInvoiceOption
showCloneInvoiceOption
showPayInvoiceOption
showFinalizeInvoiceOption
showDeleteOption={false}
showVoidInvoiceOption={true}
showApproveInvoiceOption={false}
onEditOptionClick={onEditInvoice}
/>
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the invoice details to be displayed.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide edit option, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide delete option, default value is
true
.
- Name
showSendInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide send invoice option, default value is
true
.
- Name
showCloneInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide clone invoice, default value is
true
.
- Name
showApproveInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide approve invoice option, default value is
true
.
- Name
showPayInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide pay invoice button, default value is
true
.
- Name
showVoidEstimateOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide void invoice option, default value is
true
.
- Name
showFinalizeInvoiceOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide finalize invoice button, default value is
true
.
Events
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when invoice edit option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in invoice details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in invoice details component.
<InvoiceDetails id="invoice_id">
{{
header: () => (
<div className="flex justify-between">
<h1 className="text-2xl">Invoice Details</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
Edit
</button>
</div>
</div>
),
breadcrumb: (invoice: any) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Invoices" />
<BreadcrumbItem title={invoice?.invoice_number} active />
</Breadcrumb>
),
}}
</InvoiceDetails>
Invoice Create
This component serves as an interface for users to input and submit information essential for generating a new invoice. It handles the UI elements & data validation to create invoices within your application.
Preview
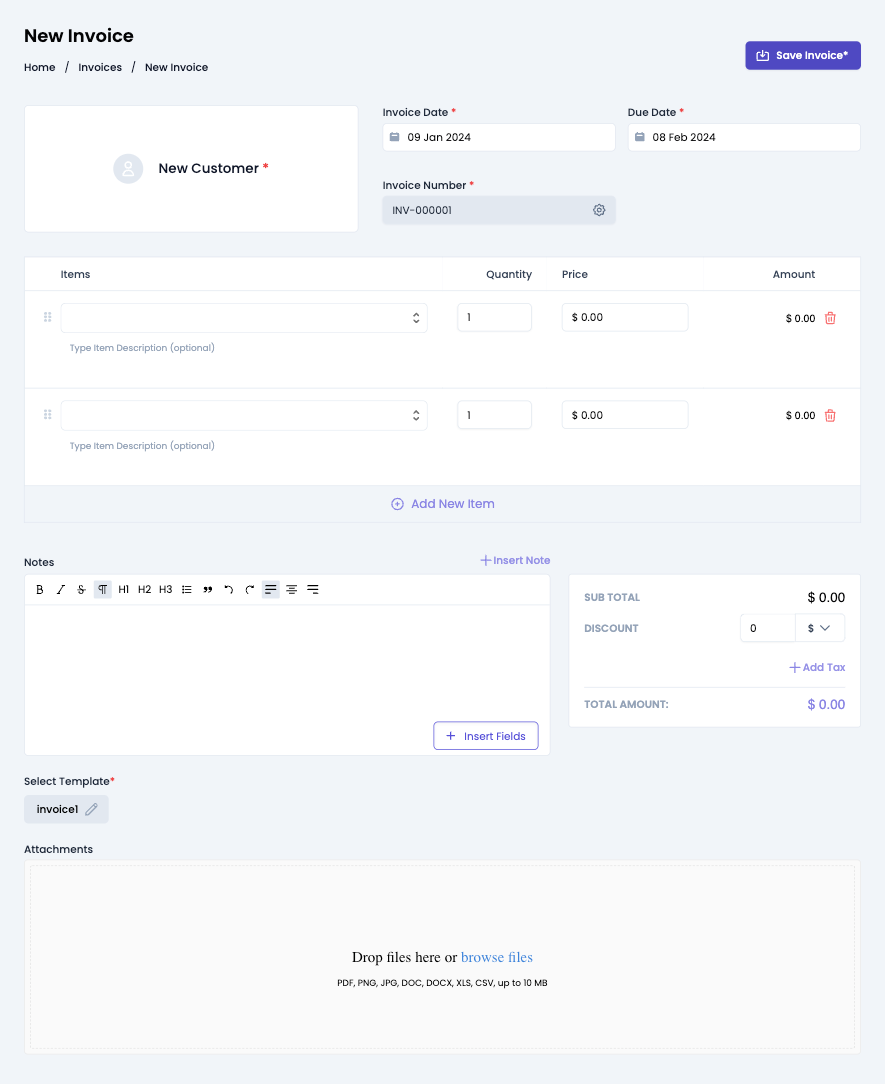
Usage
Renders a form for creating a new invoice. Use in the InvoiceCreate component in your application as shown:
InvoiceCreate.tsx
import { InvoiceCreate } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<InvoiceCreate showDetailOnSuccess={false} onCreate={onInvoiceCreate} />
</>
)
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice details component after invoice created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- Field Type
- Description
Triggered when an invoice is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in invoice create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in invoice create component.
<InvoiceCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Invoice</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Invoices" />
<BreadcrumbItem title="New Invoice" active />
</Breadcrumb>
),
}}
</InvoiceCreate>
Invoice Edit
This component renders a form for editing a pre-existing invoice. It encompasses functionalities tailored to allow users to update specific details of an invoice.
Preview
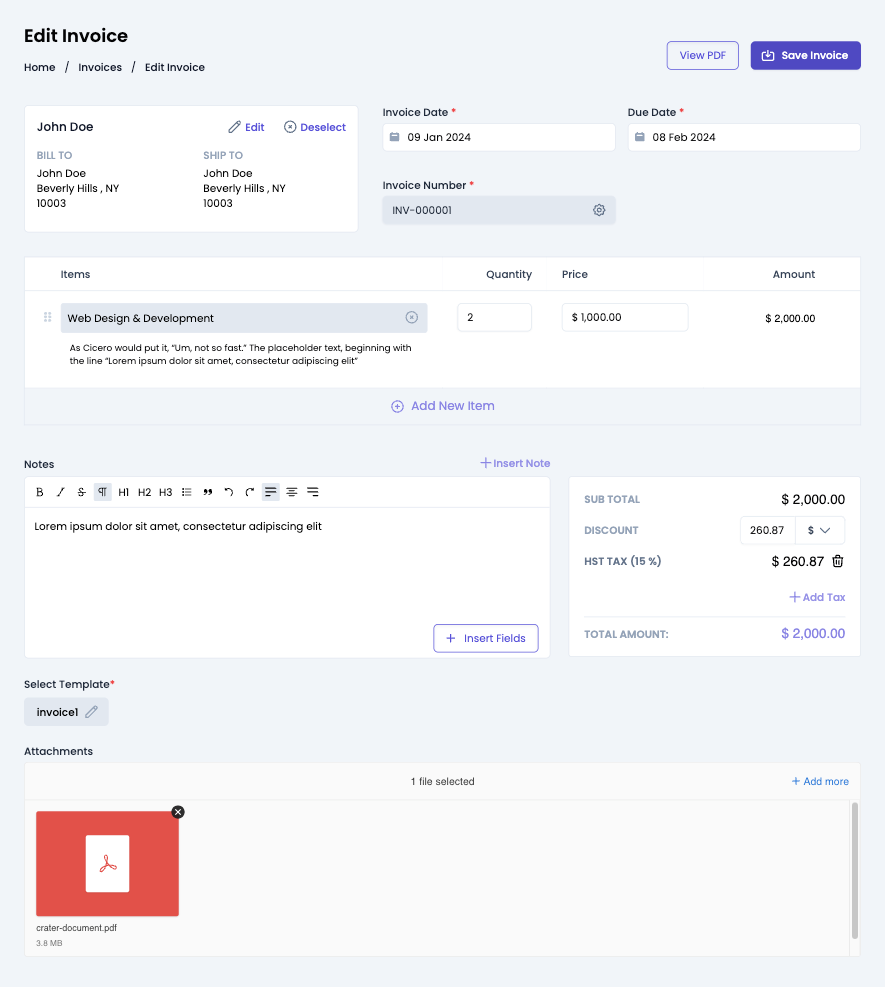
Usage
Renders a form for editing an existing invoice. Use in the InvoiceEdit component in your application as shown below:
InvoiceEdit.tsx
import { InvoiceEdit } from '@craterapp/react-sdk';
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<InvoiceEdit id="INVOICE_ID" showDetailOnSuccess={false} onUpdate={onInvoiceEdit} />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the invoice.
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide invoice details component after invoice edited successfully.
Events
- Name
onUpdate
- Type
- Field Type
- Description
Triggered when the invoice is updated successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in invoice edit component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in invoice edit component.
<InvoiceEdit id="INVOICE_ID">
{{
header: (invoice) => (
<div className="flex items-center justify-between">
<h1>Edit Invoice</h1>
</div>
),
breadcrumb: (invoice) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Invoices" />
<BreadcrumbItem title="Edit Invoice" active />
</Breadcrumb>
),
}}
</InvoiceEdit>
Invoice Approve
This component serves as an interface for users to approve and schedule payment for invoice. It handles the UI elements & data validation to create invoices within your application.
Preview
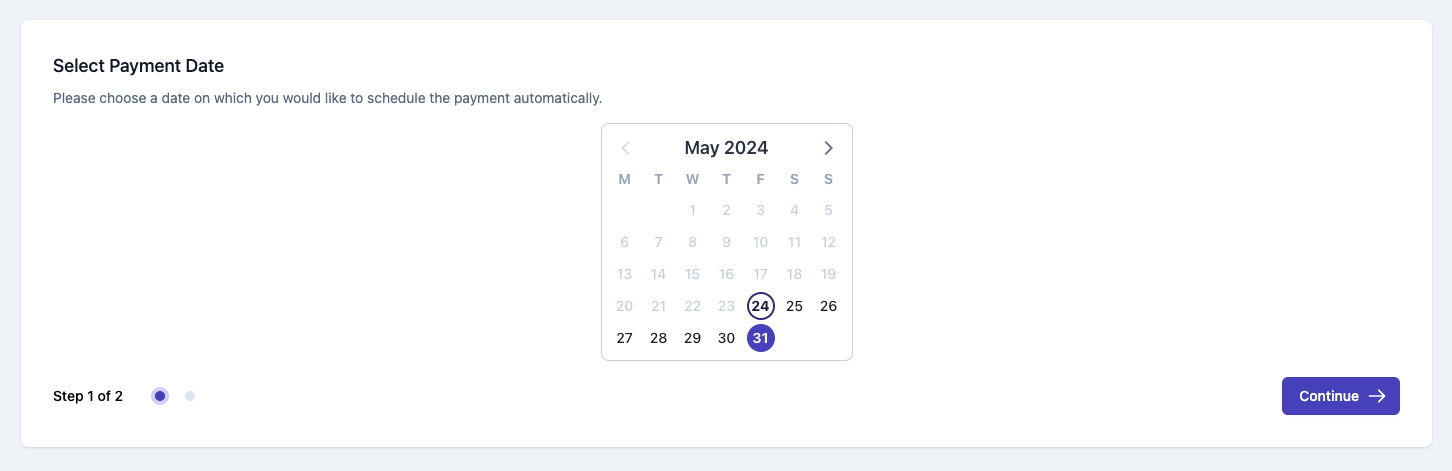
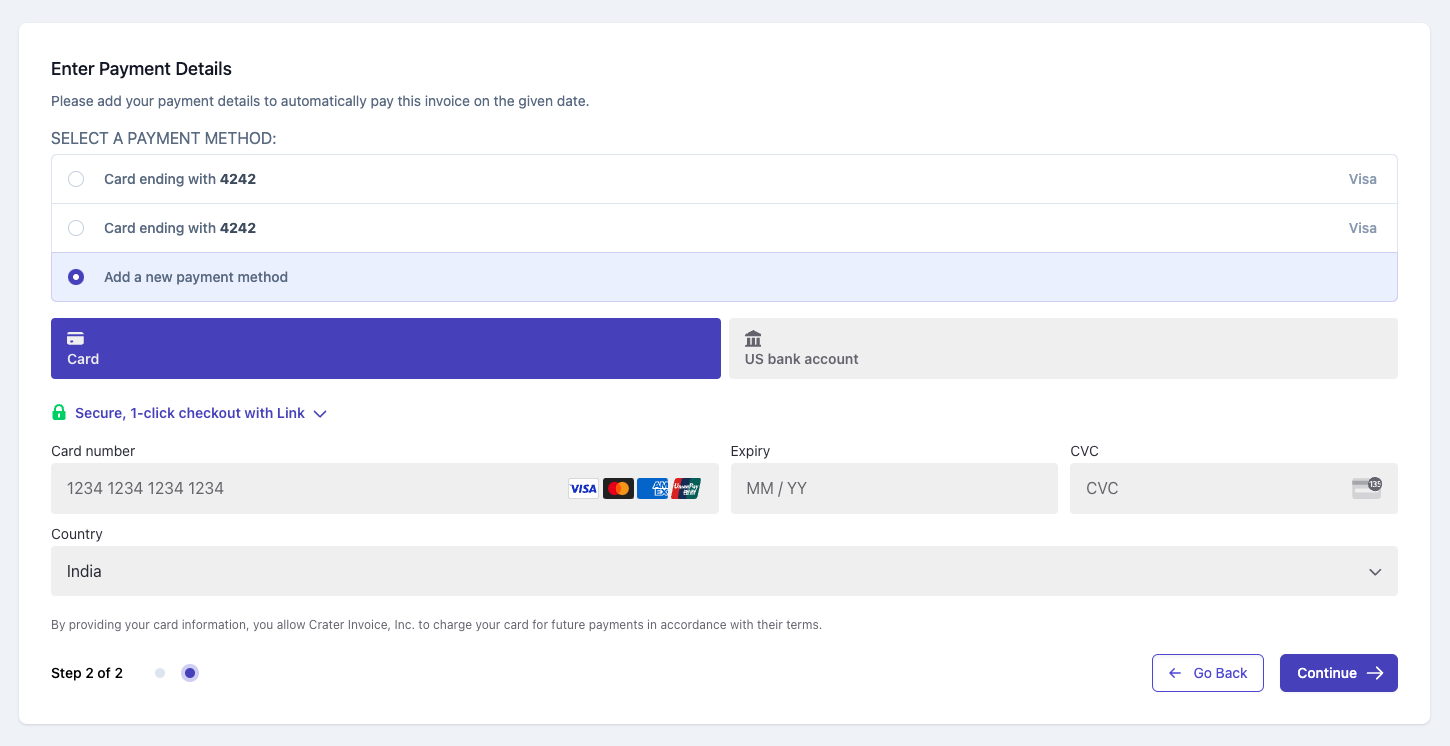
Usage
To approve existing invoice and schedule future payment, use the InvoiceApprove component with the invoice-id prop as shown:
InvoiceApprove.tsx
import { InvoiceApprove } from '@crater/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<InvoiceApprove id="INVOICE_ID" onSuccess={onSuccess} onError={onError} />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the invoice.
Events
- Name
onSuccess
- Type
- Field Type
- Description
Triggered when the invoice approved successfully.
- Name
onError
- Type
- Field Type
- Description
Triggered if the invoice approve has some issue.
Recurring Invoice Table
This component displays a table of all recuring invoices. The component shows each recurring invoice's repeat interval, total and status as well as other required details.
Preview
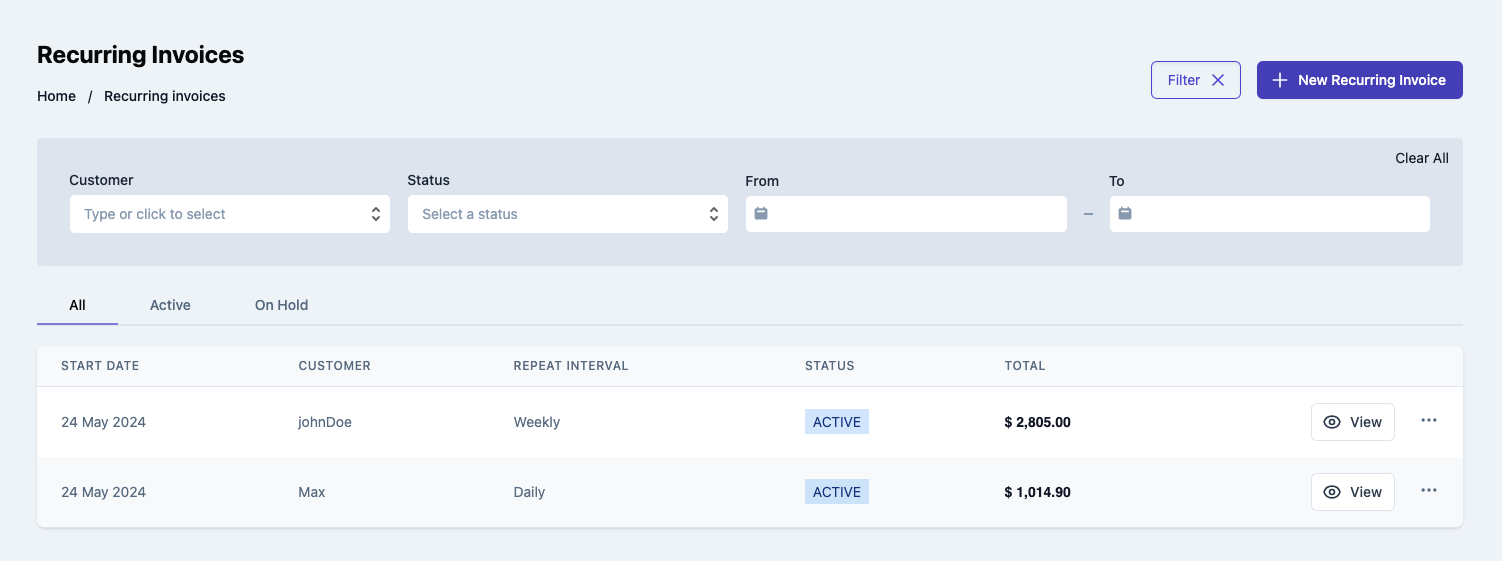
Usage
Renders a table of recurring invoice. Use the RecurringInvoiceTable component in your application as shown below:
RecurringInvoiceTable.tsx
import { RecurringInvoiceTable } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<RecurringInvoiceTable
showFilters
showTableTitle
showTabs
showEditOption
showDeleteOption
showViewOption
showCreateButton={false}
onCreateButtonClick={onRecurringInvoiceCreate}
onEditOptionClick={onRecurringInvoiceEdit}
onViewOptionClick={onRecurringInvoiceView}
/>
</>
)
Props
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button, default value is
true
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with 'customer_id', 'status', 'from_date', 'to_date' key and its value for applying filter.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice table title, default value is
true
.
- Name
showTabs
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice status tabs, default value is
true
.
- Name
showCreateButton
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice create button, default value is
true
.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice edit option, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice delete option, default value is
true
.
- Name
showViewOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice view option, default value is
true
.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['start_date', 'name', 'repeat_interval', 'status', 'total', 'view_action', 'actions']
Example:
If you pass['start_date', 'name', 'repeat_interval',]
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<RecurringInvoiceTable columns="[{ key: 'name', label: 'Customer Name', thClass: 'table-cell', tdClass: 'table-cell', sortable: true, hidden: false, }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<RecurringInvoiceTable columns="[ 'name', { key: 'status', label: 'Status', sortable: false } ]" />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a recurring invoice table row is clicked.
- Name
onCreateButtonClick
- Type
- Field Type
- Description
Triggered when recurring invoice create button clicked.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when recurring invoice view option clicked from options menu.
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when recurring invoice edit option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in recurring invoice table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in recurring invoice table component.
<RecurringInvoiceTable>
{{
header: () => (
<div className="flex justify-between">
<h1 className="text-2xl">Recurring Invoices</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
New Recurring Invoice
</button>
</div>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Recurring Invoices" active />
</Breadcrumb>
),
}}
</RecurringInvoiceTable>
Recurring Invoice Details
This component renders the interface for displaying an existing recurring invoice. It also allows performing various actions on the recurring invoice such as editing and deleting it.
Preview
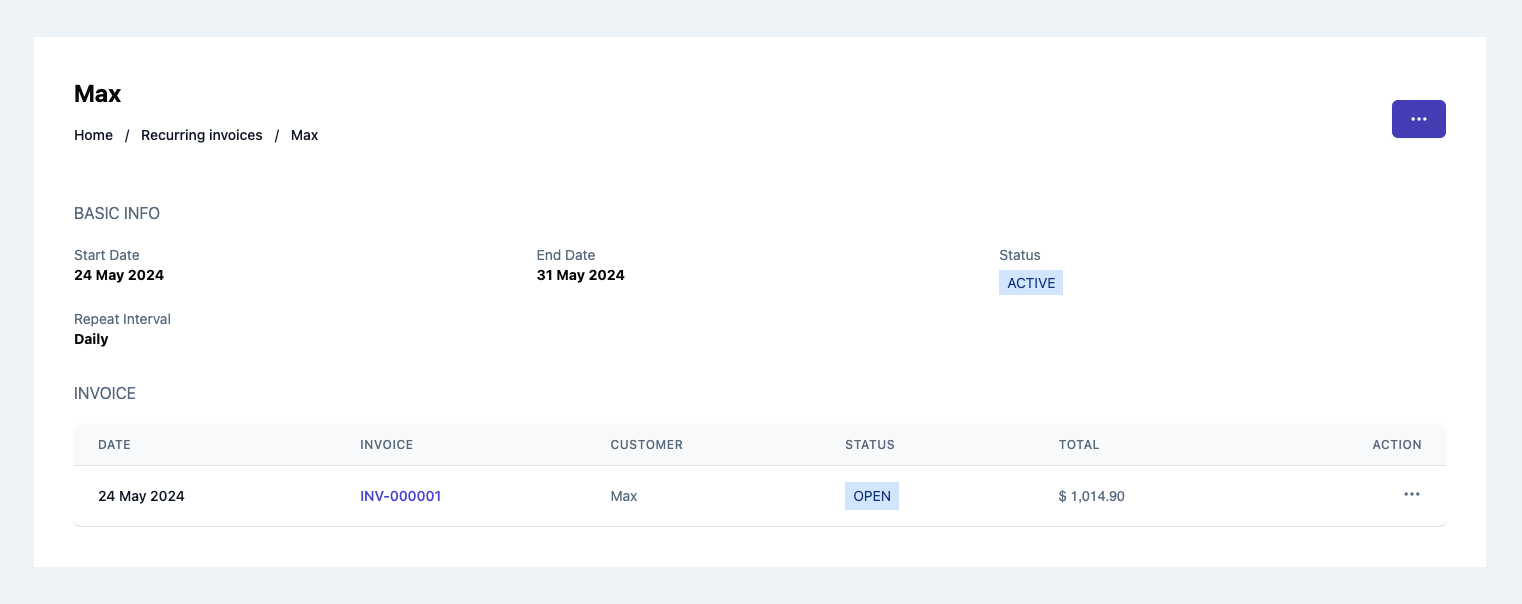
Usage
To view details of an existing recurring invoice, use the RecurringInvoiceDetails component with the id prop as shown:
RecurringInvoiceDetails.tsx
import { RecurringInvoiceDetails } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<RecurringInvoiceDetails
id="RECURRING_INVOICE_ID"
showEditOption
showDeleteOption
showEditOption
showViewOption
showSendInvoiceOption
showCloneInvoiceOption
showDeleteOption={false}
showVoidInvoiceOption={false}
onInvoiceRowClick={onInvoiceRowClick}
onInvoiceEditOptionClick={onInvoiceEditOptionClick}
onInvoiceViewOptionClick={onInvoiceRowClick}
/>
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the recurring invoice details to be displayed.
- Name
showEditOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide edit recurring invoice option, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide delete recurring invoice option, default value is
true
.
- Name
showDeleteOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide delete invoice option, default value is
true
.
- Name
showViewOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide view invoice option, default value is
true
.
- Name
showInvoiceEditOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide edit invoice option, default value is
true
.
- Name
showInvoiceViewOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide view invoice option, default value is
true
.
- Name
showInvoiceSendOption
- Type
- boolean
- Field Type
optional
- Description
This is a optional prop that accepts the boolean value to show / hide send invoice option, default value is
true
.
Events
- Name
onEditOptionClick
- Type
- Field Type
- Description
Triggered when recurring invoice edit option clicked.
- Name
onInvoiceRowClick
- Type
- Field Type
- Description
Triggered when invoice edit option clicked.
- Name
onInvoiceEditOptionClick
- Type
- Field Type
- Description
Triggered when invoice edit option clicked.
- Name
onInvoiceViewOptionClick
- Type
- Field Type
- Description
Triggered when invoice view option clicked.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in recurring invoice details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in recurring invoice details component.
<RecurringInvoiceDetails>
{{
header: (recurringInvoice) => {
return (
<div className="flex justify-between">
<h1 className="text-2xl">Recurring Invoice Details</h1>
<div className="flex items-center">
<button type="button" className="mr-2">
Edit
</button>
</div>
</div>
)
},
breadcrumb: (recurringInvoice: any) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Recurring Invoices" />
<BreadcrumbItem title={recurringInvoice?.customer?.name} active />
</Breadcrumb>
),
}}
</RecurringInvoiceDetails>
Recurring Invoice Create
This component serves as an interface for users to input and submit information essential for generating a new rucurring invoice. It handles the UI elements & data validation to create recurring invoices within your application.
Preview
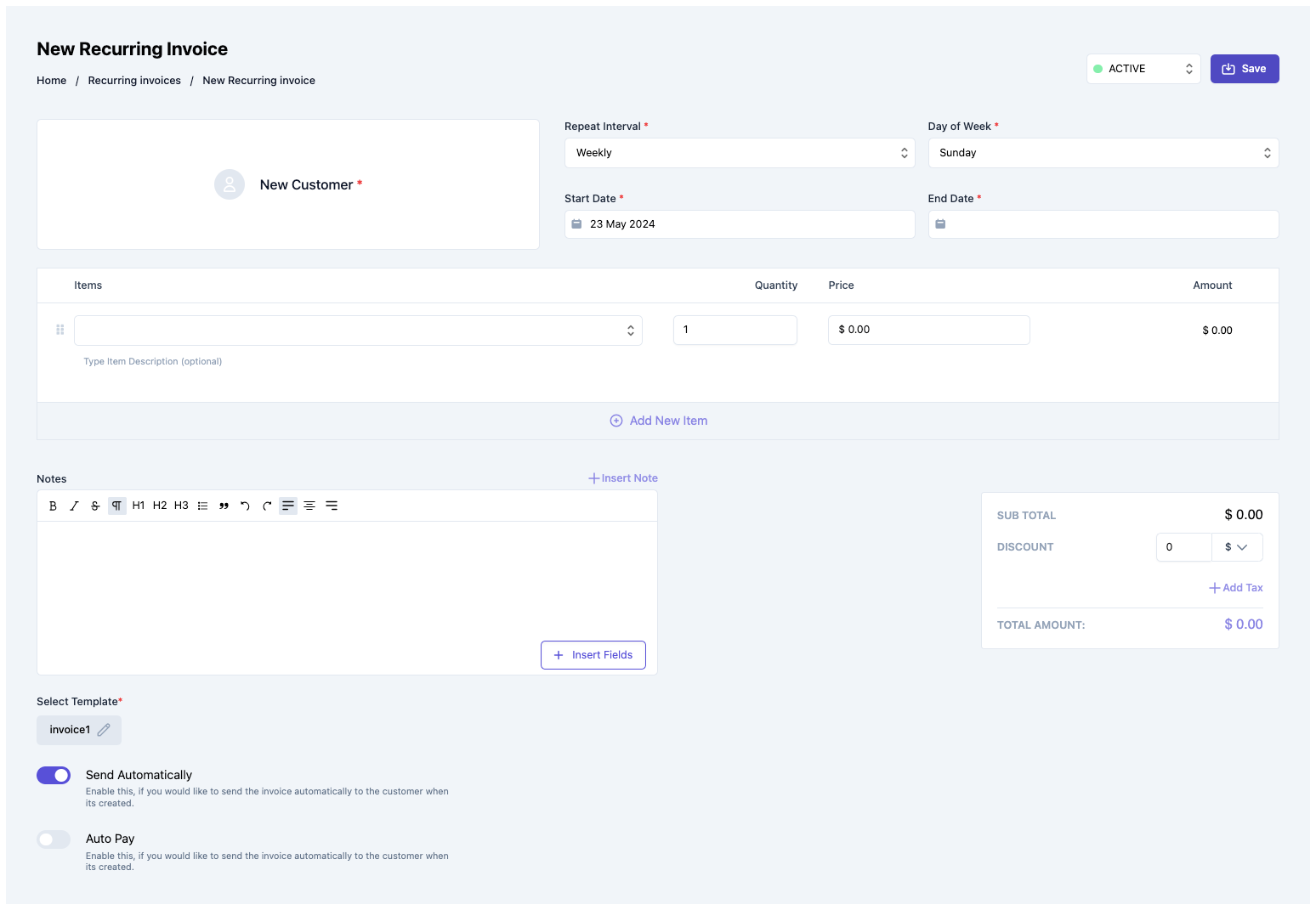
Usage
Renders a form for creating a new recurring invoice. Use the RecurringInvoiceCreate component in your application as shown below:
RecurringInvoiceCreate.tsx
import { RecurringInvoiceCreate } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<RecurringInvoiceCreate showDetailOnSuccess={false} onCreate={onRecurringInvoiceCreate} />
</>
)
Props
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice details component after recurring invoice created successfully, default value is
true
.
Events
- Name
onCreate
- Type
- function
- Field Type
- Description
Triggered when a recurring invoice is created successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in recurring invoice create component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in recurring invoice create component.
<RecurringInvoiceCreate>
{{
header: () => (
<div className="flex items-center justify-between">
<h1>New Recurring Invoic</h1>
</div>
),
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Recurring Invoice" />
<BreadcrumbItem title="New Recurring Invoice" active />
</Breadcrumb>
),
}}
</RecurringInvoiceCreate>
Recurring Invoice Edit
This component renders a form for editing a pre-existing recurring invoice. It encompasses functionalities tailored to allow users to update specific details of an recurring invoice.
Preview
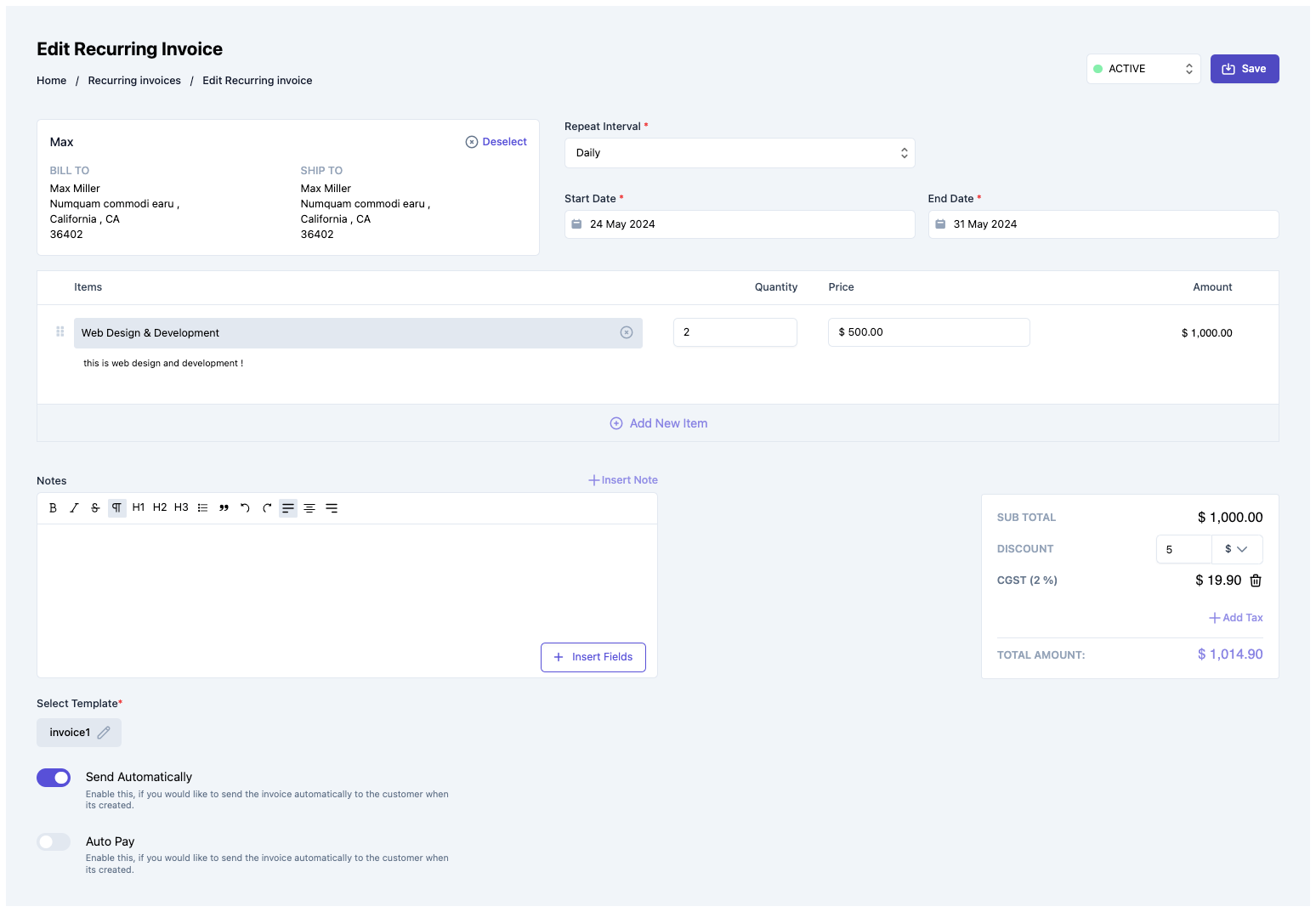
Usage
Renders a form for editing an existing recurring invoice. Use the RecurringInvoiceEdit component in your application as shown below:
RecurringInvoiceEdit.tsx
import { RecurringInvoiceEdit } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<RecurringInvoiceEdit
id="RECURRING_INVOICE_ID"
showDetailOnSuccess={false}
onUpdate={onRecurringInvoiceEdit}
/>
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the recurring invoice.
- Name
showDetailOnSuccess
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide recurring invoice details component after recurring invoice updated successfully, default value is
true
.
Events
- Name
onUpdate
- Type
- Field Type
- Description
Triggered when the recurring invoice is updated successfully.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in recurring invoice edit component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in recurring invoice edit component.
<RecurringInvoiceEdit id="RECURRING_INVOICE_ID">
{{
header: (recurringInvoice) => (
<div className="flex items-center justify-between">
<h1>Edit Recurring Invoice</h1>
</div>
),
breadcrumb: (recurringInvoice) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Recurring Invoices" />
<BreadcrumbItem title="Edit Recurring Invoice" active />
</Breadcrumb>
),
}}
</RecurringInvoiceEdit>
Payment Table
This component displays a table of all payments. The component shows each payment's payment date, business name and payment mode as well as other required details.
Preview
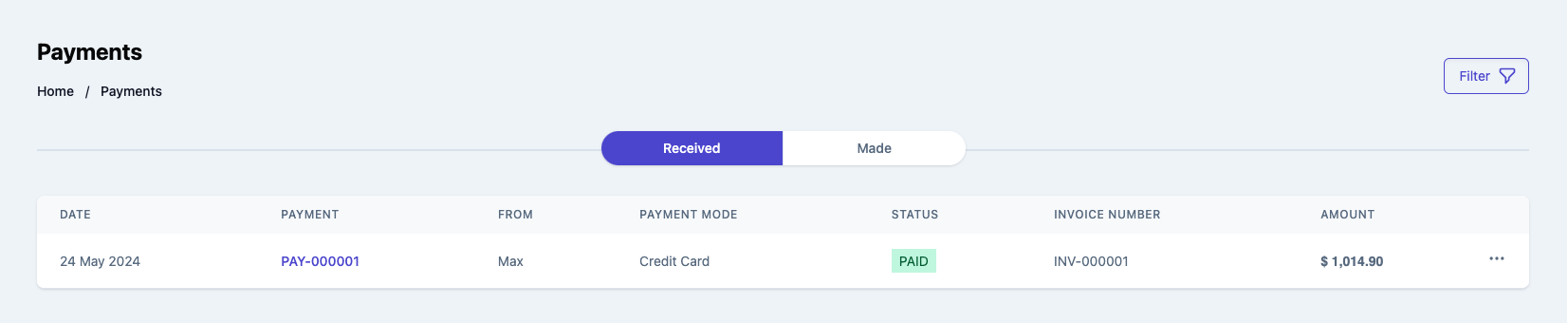
Usage
Renders a table of payments. Use in the PaymentTable component in your application as shown:
PaymentTable.tsx
import { PaymentTable } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<PaymentTable
showFilters
showViewOption
onRowClick={onRowClick}
onViewOptionClick={onPaymentView}
/>
</>
)
Props
- Name
showFilters
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide filters button, default value is
true
.
- Name
applyFilters
- Type
- object
- Field Type
optional
- Description
This prop accepts object with
customer_business_id
,payment_number
,payment_mode
,vendor_business_id
key and its value for applying filter.
- Name
showTableTitle
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide payment table title, default value is
true
.
- Name
showViewOption
- Type
- boolean
- Field Type
optional
- Description
This prop accepts boolean value to show / hide payment delete option in table, default value is
true
.
- Name
columns
- Type
- array
- Field Type
optional
- Description
This prop accepts array to customize columns of table. you can pass columns key which you want to show in table.
Available keys:
['payment_date', 'business_name', 'payment_mode', 'status', 'amount', 'invoice_number', 'actions']
Example:
If you pass['business_name', 'payment_mode', 'status']
, the table will only display these columns.Additionally, you can pass an array of objects with available keys (
'key', 'label', 'thClass', 'tdClass', 'sortable'
) to override the default properties of a column.<PaymentTable columns="[{ key: 'business_name', label: 'Name', thClass: 'text-left', tdClass: 'text-left', sortable: true, }]" />
You can also pass a combination of both arrays and objects to customize the columns of the table.
<PaymentTable columns="[ 'business_name', { key: 'status', label: 'Status', sortable: false } ]" />
Events
- Name
onRowClick
- Type
- Field Type
- Description
Triggered when a payment table row is clicked.
- Name
onViewOptionClick
- Type
- Field Type
- Description
Triggered when payment view option clicked from options menu.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in payment table component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in payment table component.
<PaymentTable>
{{
header: () => <h1 className="text-2xl">Payments</h1>,
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Payments" active />
</Breadcrumb>
),
}}
</PaymentTable>
Payment Details
This component renders the interface for displaying an existing payment.
Preview
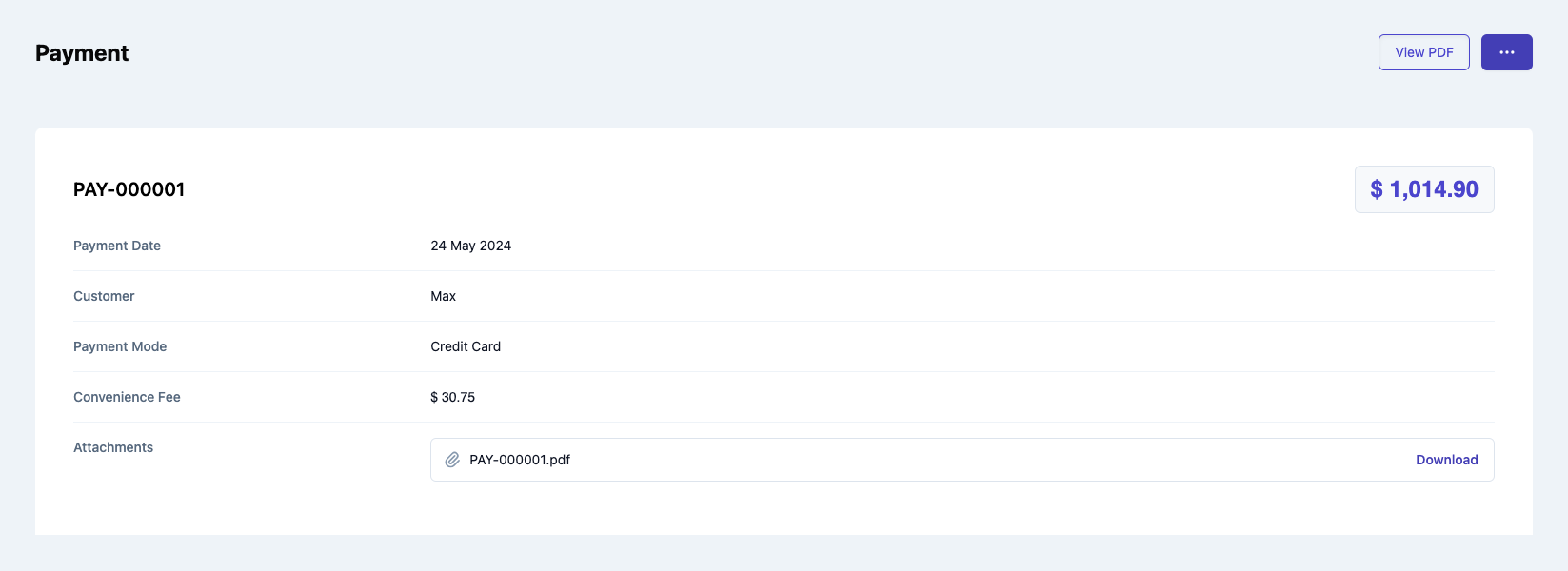
Usage
Use the PaymentDetails component to view details of an existing payment.
PaymentDetails.tsx
import { PaymentDetails } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<PaymentDetails id="PAYMENT_ID" />
</>
)
Props
- Name
id
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the ID of the payment details to be displayed.
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in payment details component.
- Name
breadcrumb
- Type
- Field Type
- Description
This slot allows you to add custom breadcrumb in payment details component.
<PaymentDetails id="PAYMENT_ID">
{{
header: (payment) => {
return <h1 className="text-2xl">Payment details</h1>
},
breadcrumb: (payment: any) => (
<Breadcrumb>
<BreadcrumbItem title="Home" />
<BreadcrumbItem title="Payments" />
<BreadcrumbItem title={payment?.payment_number} active />
</Breadcrumb>
),
}}
</PaymentDetails>
Integration Settings
This component renders a list of integrations available on the given business. Users can choose to connect any of the given integration partners to automatically sync their data.
Preview
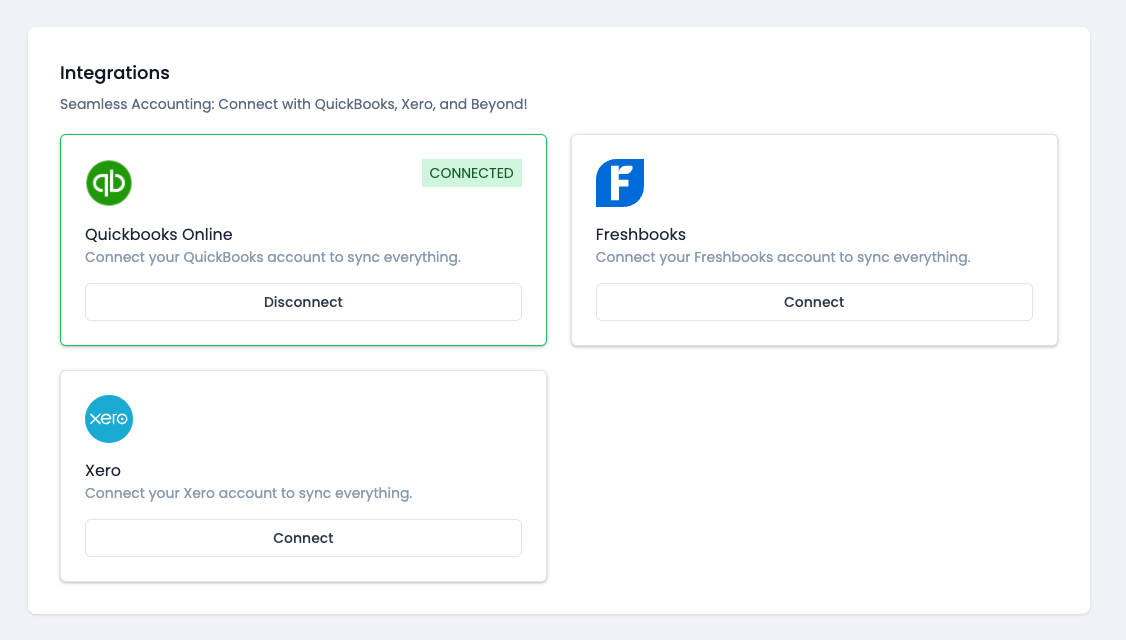
Usage
Import the IntegrationSettings component and use it in your application as shown below:
IntegrationSettings.tsx
import { IntegrationSettings } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<IntegrationSettings />
</>
)
Bread Crumb
This component is used for the breadcrumb on your page, contains a series of clickable links that represent the logical hierarchy of a user's journey within a website.
Preview
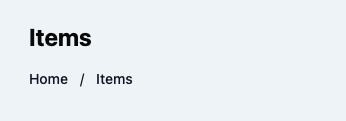
Usage
Renders a Breadcrumb. Use the Breadcrumb component in your application as shown below:
EstimateTable.tsx
import { EstimateTable, Breadcrumb, BreadcrumbItem } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateTable>
{{
breadcrumb: () => (
<Breadcrumb>
<BreadcrumbItem title="Home" onClick={() => {}} />
<BreadcrumbItem title="Estimates" active />
</Breadcrumb>
),
}}
</EstimateTable>
</>
)
Additionally, you can get the props like,
EstimateDetails.tsx
import { EstimateDetails, Breadcrumb, BreadcrumbItem } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<EstimateDetails>
{{
breadcrumb: (estimate) => (
<Breadcrumb>
<BreadcrumbItem title="Home" onClick={() => {}} />
<BreadcrumbItem title="Estimates" onClick={() => {}} />
<BreadcrumbItem title={estimate?.estimate_number} active />
</Breadcrumb>
),
}}
</EstimateDetails>
</>
)
Props
- Name
title
- Type
- string
- Field Type
required
- Description
This is a required prop that accepts the title of the breadcrumb to be displayed.
- Name
active
- Type
- boolean
- Field Type
optional
- Description
This prop accepts a boolean value to activate the breadcrumb display.
Events
- Name
onClick
- Type
- Field Type
- Description
Triggered when a breadcrumb item is clicked.
Business Overview Line Chart Widget
This component designed to provide a comprehensive overview of key business metrics through a line chart. This component visualizes the total sales, revenue, purchases, and payments of the current business, offering a clear and dynamic representation of these critical data points.
Preview
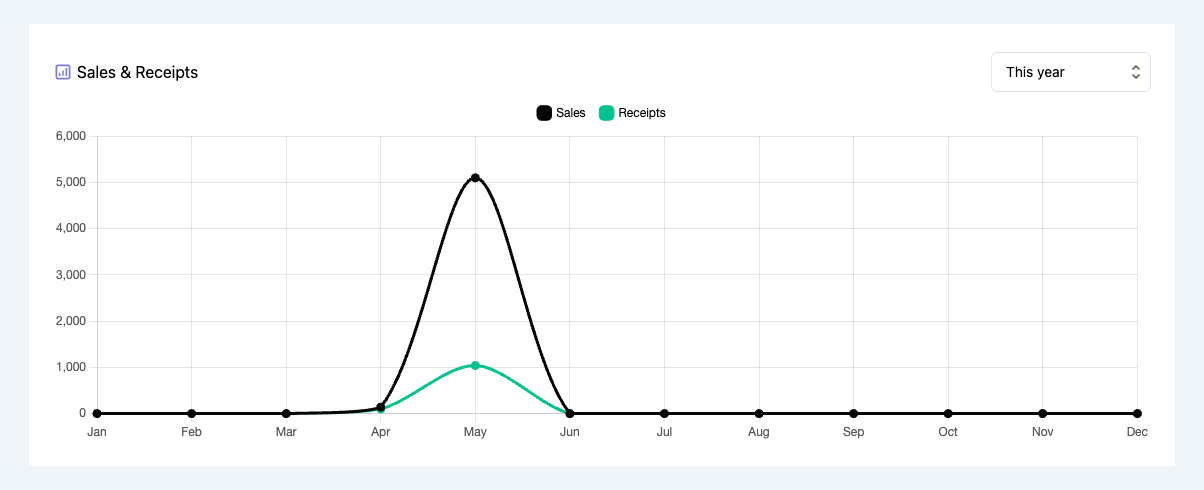
Usage
BusinessOverviewLineChartWidget.tsx
import { BusinessOverviewLineChartWidget } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<BusinessOverviewLineChartWidget
title="Sales & Receipts"
interval="monthly"
types={['payments', 'purchases']}
/>
</>
)
Props
- Name
title
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for title of the chart.
- Name
types
- Type
- array
- Field Type
optional
- Description
This is a optional prop that accepts string for type of chart to display. like if you pass
sales
, the chart will display sales details.Available types:
['sales', 'revenue', 'purchases', 'payments']
- Name
counterBusinessId
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts id of counter business for display chart with current business.
- Name
startDate
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for start-date in
'YYYY-MM-DD'
format.
- Name
endDate
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for end-date in
'YYYY-MM-DD'
format.
- Name
interval
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts interval for the chart data, which can be "monthly", "quarterly", or "yearly". This prop determines how the data points are aggregated and displayed, default value is
monthly
.Available interval:
['monthly', 'quarterly', 'yearly']
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in line chart widget component.
- Name
footer
- Type
- Field Type
- Description
This slot allows you to add additonal footer in line chart widget component.
<BusinessOverviewLineChartWidget
className="bg-white px-6 py-6 rounded-md"
interval="monthly"
startDate={startDate}
endDate={endDate}
>
{{
header: (chartData) => (
<div className="flex flex-col sm:flex-row justify-between">
<h6 className="flex items-center">
Business Overview
</h6>
<div className="my-5 sm:my-0 w-full sm:w-40 h-10">
<DatePicker
value={selectedYear}
options={years}
/>
</div>
</div>
),
footer: (chartData) => {
return <div className="my-2">Payments: {chartData?.payments}</div>
},
}}
</BusinessOverviewLineChartWidget>
Business Overview vertical Bar Chart Widget
This component designed to provide a comprehensive overview of key business metrics through a vertical bar chart. This component visualizes the total sales, revenue, purchases, and payments of the current business, offering a clear and dynamic representation of these critical data points.
Preview
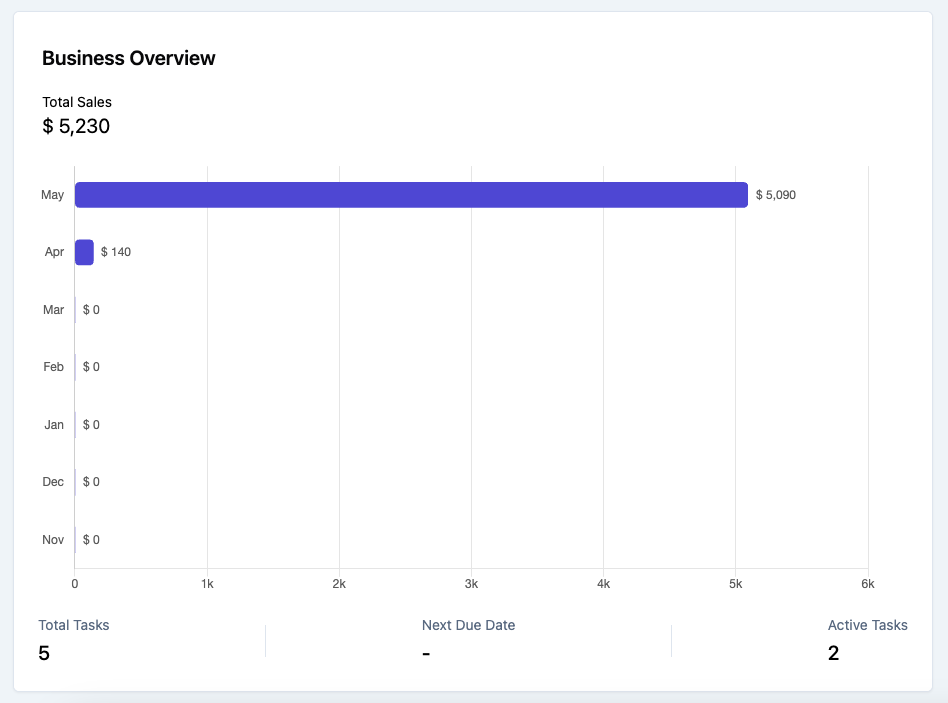
Usage
BusinessOverviewVerticalBarChartWidget.tsx
import { BusinessOverviewVerticalBarChartWidget } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<BusinessOverviewVerticalBarChartWidget
title="Business Overview"
type="sales"
interval="monthly"
/>
</>
)
Props
- Name
title
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for title of the chart.
- Name
type
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for type of chart to display. like if you pass
sales
, the chart will display sales chart.Available types:
['sales', 'revenue', 'purchases', 'payments']
- Name
counterBusinessId
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts id of counter business for display chart with current business.
- Name
startDate
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for start-date in
'YYYY-MM-DD'
format.
- Name
endDate
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for end-date in
'YYYY-MM-DD'
format.
- Name
interval
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts interval for the chart data, which can be "monthly", "quarterly", or "yearly". This prop determines how the data points are aggregated and displayed.
Available interval:
['monthly', 'quarterly', 'yearly']
Children
- Name
header
- Type
- Field Type
- Description
This slot allows you to add custom header in bar chart widget component.
- Name
footer
- Type
- Field Type
- Description
This slot allows you to add additonal footer in bar chart widget component.
Example:
BusinessOverviewVerticalBarChartWidget.tsx
import { BusinessOverviewVerticalBarChartWidget } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<BusinessOverviewVerticalBarChartWidget
title="Business Overview"
startDate={dateRange.startDate}
endDate={dateRange.endDate}
>
{{
header: (chartData) => (
<div className="flex flex-col sm:flex-row justify-between">
<h6 className="flex items-center">Business Overview</h6>
<div className="my-5 sm:my-0 w-full sm:w-40 h-10">
<DatePicker value={selectedYear} options={years} />
</div>
</div>
),
footer: (chartData) => {
return (
<div className="flex gap-5 flex-col mt-4">
<div className="flex flex-col">
<p className="text-sm font-normal text-gray-500">Total Revenue</p>
<h3 className="text-xl font-medium mt-1">{chartData.revenue}</h3>
</div>
<span className="h-8 w-px bg-gray-200 hidden sm:flex" />
<div className="flex flex-col">
<p className="text-sm font-normal text-gray-500">Next Due Date</p>
<h3 className="text-xl font-medium mt-1">-</h3>
</div>
<span className="h-8 w-px bg-gray-200 hidden sm:flex" />
<div className="flex flex-col">
<p className="text-sm font-normal text-gray-500">Active Tasks</p>
<h3 className="text-xl font-medium mt-1">-</h3>
</div>
</div>
)
},
}}
</BusinessOverviewVerticalBarChartWidget>
</>
)
Platform Overview Chart Widget
This component designed to provide a comprehensive overview of multiple businesses' data in a single chart. This component allows users to select a specific business to view its data, offering a versatile tool for comparing and analyzing different businesses' performances.
Platform access token is required to access this component, check the quickstart guide to get the platform access token.
Preview
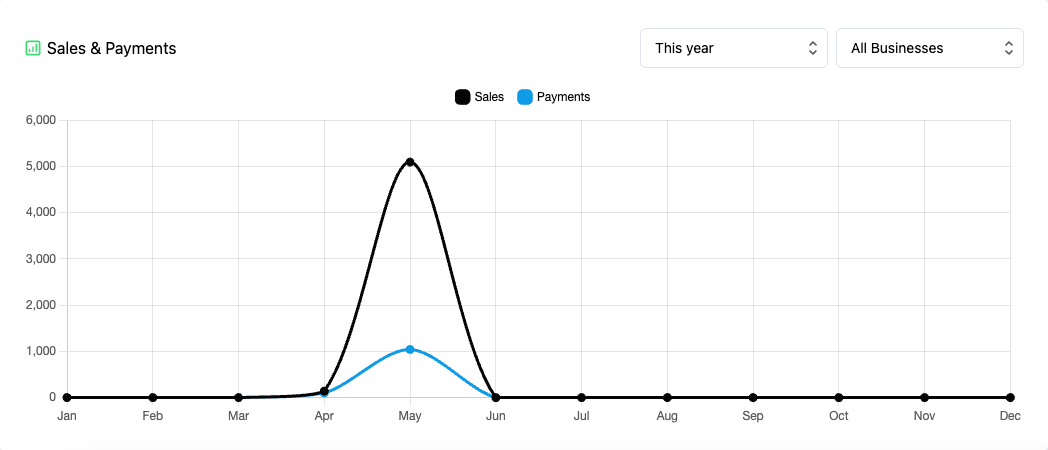
Usage
PlatformOverviewChartWidget.tsx
import { PlatformOverviewChartWidget } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<PlatformOverviewChartWidget allOptionLabel="All Businesses" />
</>
)
Props
- Name
allOptionLabel
- Type
- string
- Field Type
optional
- Description
This is a optional prop that accepts string for lable to display in dropdown for
all businesses
option. like if you passAll
, the dropdown will showAll
as option instead ofAll Businesses
option.
Children
- Name
default
- Type
- Field Type
- Description
You can use default slot to add custom content in platform overview chart widget.
Example:
PlatformOverviewChartWidget.tsx
import { PlatformOverviewChartWidget } from '@craterapp/react-sdk'
...
// NOTE: This component must be rendered within the CraterProvider wrapper
return (
<>
<PlatformOverviewChartWidget allOptionLabel="All Businesses">
<div>
<h1>Custom Content</h1>
</div>
</PlatformOverviewChartWidget>
</>
)